短进程优先算法代码C语言
时间: 2024-09-06 11:07:41 浏览: 50
短进程优先(Scheduling)算法是一种作业调度策略,它优先处理那些剩余运行时间较短的进程,以提高系统的响应时间和整体效率。在C语言中,实现这种算法通常涉及到以下几个步骤:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 定义进程结构体
typedef struct {
int pid; // 进程ID
float burst_time; // 进程运行时间
} Process;
// 比较进程剩余运行时间函数
int compare_processes(const void *a, const void *b) {
Process *p1 = (Process *) a;
Process *p2 = (Process *) b;
return p1->burst_time - p2->burst_time;
}
void sjf scheduling(Process processes[], int n) {
qsort(processes, n, sizeof(Process), compare_processes);
int t = 0; // 时间
for (int i = 0; i < n; i++) {
while (t + processes[i].burst_time > processes[i].pid) {
printf("CPU is idle at time %d\n", t);
t += 1;
}
printf("Process %d starts at time %d and finishes at time %d\n",
processes[i].pid, t, t + processes[i].burst_time);
t += processes[i].burst_time;
}
}
int main() {
Process processes[] = { {1, 5}, {2, 10}, {3, 3}, {4, 7} }; // 示例进程列表
int n = sizeof(processes) / sizeof(processes[0]);
printf("SJF Scheduling:\n");
sjf(processes, n);
return 0;
}
```
这个简单的C程序首先定义了一个进程结构体,然后实现了一个比较函数`compare_processes`用于对进程按照剩余运行时间排序。`sjf scheduling`函数实现了短进程优先调度逻辑,主函数则创建了几个进程实例并调用了调度函数。
阅读全文
相关推荐
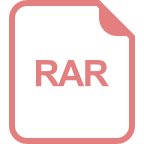
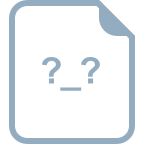
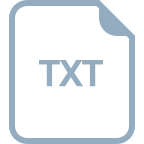





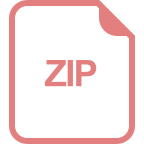
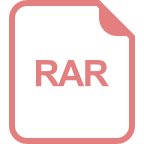
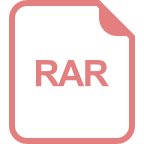
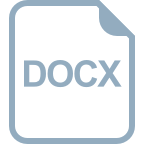
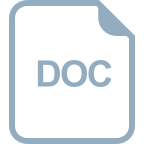



