进程调度算法-短进程优先调度模拟用C语言写代码
时间: 2024-11-28 12:27:40 浏览: 12
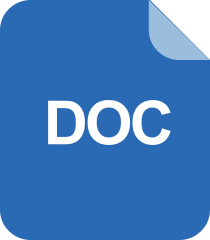
使用动态优先权的进程调度算法的模拟

在C语言中,我们可以创建一个简单的短进程优先调度(Shortest Job First, SJF)算法的模拟程序。这个算法的基本思想是优先处理完成时间最短的任务。以下是使用C语言的一个基本示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
typedef struct {
int pid; // 进程ID
float burst_time; // 进程运行时间
} Process;
// 比较函数,用于进程队列排序
int compare(const void* a, const void* b) {
return ((Process*)a)->burst_time - ((Process*)b)->burst_time;
}
void sjf_scheduling(Process processes[], int n) {
qsort(processes, n, sizeof(Process), compare); // 对进程按burst_time排序
int t = 0; // 当前时间
for (int i = 0; i < n; ++i) { // 遍历所有进程
while (processes[i].burst_time > t) { // 当前进程未完成
printf("Processing PID %d with burst time %f\n", processes[i].pid, processes[i].burst_time);
t += processes[i].burst_time; // 更新当前时间
}
printf("PID %d completed at time %f\n", processes[i].pid, t);
}
}
int main() {
int num_processes;
printf("Enter the number of processes: ");
scanf("%d", &num_processes);
Process processes[num_processes];
for (int i = 0; i < num_processes; ++i) {
printf("Enter PID and burst time for process %d: ", i + 1);
scanf("%d%f", &processes[i].pid, &processes[i].burst_time);
}
sjf_scheduling(processes, num_processes);
return 0;
}
```
在这个代码中,用户输入每个进程的ID和运行时间(burst time),然后我们通过`sjf_scheduling`函数按照这些值对进程进行排序,并模拟它们在SJF调度下的执行顺序。
阅读全文
相关推荐
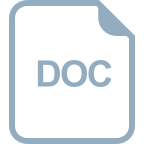
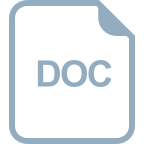


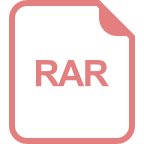
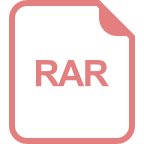
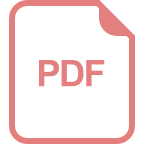
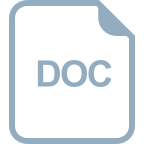
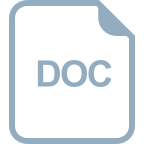








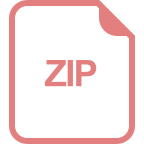