帮我用c语言写一个短进程优先的调度算法,输出能够运行的完整代码
时间: 2024-10-29 09:20:52 浏览: 49
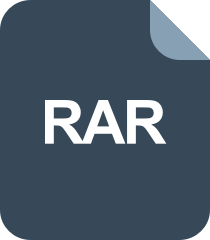
【C语言源代码】 操作系统-短进程优先-进程调度算法
在C语言中,实现短进程优先(Shortest Job First, SJF)调度算法通常涉及到进程管理的数据结构和基本操作。由于这里是一个简化的版本,我们假设有一个任务队列和每个任务都有一个已知的执行时间。下面是一个简单的示例,我们将使用链表来存储任务:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Task {
int pid; // 进程ID
int exec_time; // 执行时间
struct Task* next;
} Task;
Task* create_task(int id, int time) {
Task* new_task = (Task*)malloc(sizeof(Task));
if (!new_task) {
printf("Memory allocation failed.\n");
return NULL;
}
new_task->pid = id;
new_task->exec_time = time;
new_task->next = NULL;
return new_task;
}
void insert_task(Task** queue, int id, int time) {
Task* new_task = create_task(id, time);
if (!new_task) return;
if (*queue == NULL) {
*queue = new_task;
} else {
Task* current = *queue;
while (current->next != NULL && current->next->exec_time < new_task->exec_time) {
current = current->next;
}
new_task->next = current->next;
current->next = new_task;
}
}
// 假设我们有个全局变量 task_queue 存储当前队列
int sjf_scheduler() {
Task* current = task_queue;
while (current != NULL) {
printf("Executing process %d with execution time %d\n", current->pid, current->exec_time);
// 模拟进程执行
sleep(current->exec_time); // 睡眠对应的时间
current = current->next;
}
return 0;
}
int main() {
Task* task_queue = NULL;
// 示例任务插入
insert_task(&task_queue, 1, 5);
insert_task(&task_queue, 2, 3);
insert_task(&task_queue, 3, 7);
// 调度
sjf_scheduler();
return 0;
}
```
注意,这个例子中并没有处理进程结束后的清理工作,也没有考虑并发环境下的线程安全问题。在实际应用中,可能会需要更复杂的数据结构和同步机制。
阅读全文
相关推荐
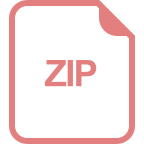


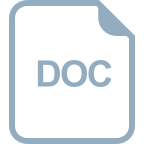












