写一个C语言版本的模拟实现短作业优先调度算法实验代码
时间: 2023-11-17 09:06:45 浏览: 58
以下是一个简单的短作业优先调度算法的C语言代码实现。
```
#include <stdio.h>
#define MAX_PROCESS 10
struct process {
int id;
int arrival_time;
int burst_time;
int waiting_time;
int turnaround_time;
int remaining_time;
int completed;
};
int main() {
int n, time = 0, total_waiting_time = 0, total_turnaround_time = 0;
struct process processes[MAX_PROCESS], *current_process = NULL;
printf("Enter the number of processes: ");
scanf("%d", &n);
// Input process info
for (int i = 0; i < n; i++) {
printf("Enter arrival time and burst time for process %d: ", i + 1);
scanf("%d %d", &processes[i].arrival_time, &processes[i].burst_time);
processes[i].id = i + 1;
processes[i].waiting_time = 0;
processes[i].turnaround_time = 0;
processes[i].remaining_time = processes[i].burst_time;
processes[i].completed = 0;
}
// Schedule processes
while (1) {
int shortest_time = 99999;
for (int i = 0; i < n; i++) {
if (processes[i].arrival_time <= time && processes[i].completed == 0) {
if (processes[i].remaining_time < shortest_time) {
shortest_time = processes[i].remaining_time;
current_process = &processes[i];
}
}
}
if (current_process == NULL) {
time++;
continue;
}
current_process->remaining_time--;
shortest_time--;
if (current_process->remaining_time == 0) {
current_process->completed = 1;
current_process->turnaround_time = time + 1 - current_process->arrival_time;
current_process->waiting_time = current_process->turnaround_time - current_process->burst_time;
total_turnaround_time += current_process->turnaround_time;
total_waiting_time += current_process->waiting_time;
current_process = NULL;
}
time++;
if (current_process == NULL && time != 0) {
break;
}
}
// Output process info
printf("\nProcess\tArrival Time\tBurst Time\tWaiting Time\tTurnaround Time\n");
for (int i = 0; i < n; i++) {
printf("%d\t\t%d\t\t%d\t\t%d\t\t%d\n", processes[i].id, processes[i].arrival_time, processes[i].burst_time, processes[i].waiting_time, processes[i].turnaround_time);
}
printf("\nAverage Waiting Time: %.2f", (float)total_waiting_time / n);
printf("\nAverage Turnaround Time: %.2f", (float)total_turnaround_time / n);
return 0;
}
```
该代码首先要求用户输入进程数量以及每个进程的到达时间和运行时间。然后,它使用短作业优先调度算法来模拟进程的执行过程,并计算出平均等待时间和平均周转时间。最后,它将所有进程的信息以表格形式输出。
相关推荐
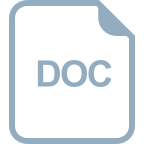














