c语言采用剥夺式优先算法,对三个进程进行模拟调度
时间: 2023-11-05 15:03:20 浏览: 80
C语言采用剥夺式优先算法可以对三个进程进行模拟调度。剥夺式优先算法是一种基于进程优先级的调度算法,它允许高优先级的进程在任何时候都可以抢占低优先级的进程的CPU资源。
对于三个进程的模拟调度,我们可以假设它们的优先级分别为P1、P2和P3,且P1的优先级最高,P3的优先级最低。在剥夺式优先算法中,每个进程根据自己的优先级轮流执行,直到一个进程被更高优先级的进程剥夺CPU资源。
开始时,P1进程优先执行,直到发生以下情况之一:
1. 当P2进程到达时,如果P2的优先级高于P1,CPU资源被剥夺给P2,P2开始执行;
2. 当P3进程到达时,如果P3的优先级高于P1,CPU资源被剥夺给P3,P3开始执行;
3. 如果P1进程执行完毕,并且P2和P3都没有到达,则空闲状态,等待下一个进程到达。
当某个进程被剥夺CPU资源后,进程的优先级可以再次被重新评估以确定下一轮的调度。比如,如果P1执行过程中,P2的优先级发生变化,高于P1,则下一轮P2可能被优先执行。
通过剥夺式优先算法调度三个进程,可以保证按照优先级高低有序执行,高优先级的进程能够尽快获得CPU资源,从而提高系统的响应速度和效率。通过编写C语言程序来模拟这个调度过程,我们可以更好地理解和研究进程调度算法的原理和机制。
相关问题
用C语言对N个进程采用动态优先权算法的进程调度
动态优先权算法是一种基于进程优先级的调度算法,它会为每个进程分配一个动态优先级,并在每个时间片结束时重新计算进程的优先级。下面是一个用C语言实现动态优先权算法的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_PROCESS 10
struct Process {
int pid; // 进程ID
int priority; // 进程优先级
int burst_time; // 进程运行时间
int waiting_time; // 进程等待时间
int turnaround_time; // 进程周转时间
};
// 计算进程的等待时间、周转时间和平均等待时间、平均周转时间
void calculate_time(struct Process *processes, int n) {
int total_waiting_time = 0;
int total_turnaround_time = 0;
for (int i = 0; i < n; i++) {
processes[i].turnaround_time = processes[i].burst_time + processes[i].waiting_time;
total_waiting_time += processes[i].waiting_time;
total_turnaround_time += processes[i].turnaround_time;
}
printf("Average waiting time: %f\n", (float)total_waiting_time / n);
printf("Average turnaround time: %f\n", (float)total_turnaround_time / n);
}
// 动态优先权调度算法
void dynamic_priority_scheduling(struct Process *processes, int n) {
int current_time = 0;
int completed_processes = 0;
while (completed_processes < n) {
int highest_priority_index = -1;
int highest_priority = -1;
// 找到当前优先级最高的进程
for (int i = 0; i < n; i++) {
if (processes[i].burst_time > 0 && processes[i].priority > highest_priority) {
highest_priority_index = i;
highest_priority = processes[i].priority;
}
}
// 如果找不到可运行的进程,则时间加一
if (highest_priority_index == -1) {
current_time++;
continue;
}
// 运行当前进程一个时间片
processes[highest_priority_index].burst_time--;
current_time++;
// 更新等待时间和优先级
for (int i = 0; i < n; i++) {
if (i != highest_priority_index && processes[i].burst_time > 0) {
processes[i].waiting_time++;
processes[i].priority--;
}
}
// 如果当前进程已经运行完毕,则更新信息
if (processes[highest_priority_index].burst_time == 0) {
completed_processes++;
processes[highest_priority_index].turnaround_time = current_time;
}
}
// 计算等待时间、周转时间和平均等待时间、平均周转时间
calculate_time(processes, n);
}
int main() {
int n;
struct Process processes[MAX_PROCESS];
printf("Enter the number of processes: ");
scanf("%d", &n);
// 输入每个进程的信息
for (int i = 0; i < n; i++) {
printf("Enter the burst time and priority of process %d: ", i + 1);
scanf("%d %d", &processes[i].burst_time, &processes[i].priority);
processes[i].pid = i + 1;
processes[i].waiting_time = 0;
}
// 运行动态优先权调度算法
dynamic_priority_scheduling(processes, n);
return 0;
}
```
在这个示例中,我们首先定义了一个 `Process` 结构体,用于存储每个进程的信息。然后,我们实现了 `calculate_time()` 函数和 `dynamic_priority_scheduling()` 函数来分别计算进程的等待时间、周转时间和平均等待时间、平均周转时间,以及运行动态优先权调度算法。
在 `dynamic_priority_scheduling()` 函数内部,我们首先定义了一个变量 `current_time` 来表示当前时间,以及一个变量 `completed_processes` 来表示已经完成的进程数。然后,在每个循环中,我们首先找到当前优先级最高的进程,并运行它一个时间片。在运行进程的同时,我们还会更新其他进程的等待时间和优先级。如果当前进程已经运行完毕,则更新它的信息并将已完成的进程数加一。最后,我们使用 `calculate_time()` 函数计算进程的等待时间、周转时间和平均等待时间、平均周转时间。
在 `main()` 函数中,我们首先输入每个进程的信息,然后运行动态优先权调度算法。
用C语言实现对N个进程采用高优先权优先进程调度算法的模拟
好的,下面是一个简单的用C语言实现对N个进程采用高优先权优先进程调度算法的模拟的示例代码。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_PROCESS 10
struct Process {
int id;
int arrival_time;
int burst_time;
int priority;
};
struct ProcessQueue {
struct Process* queue[MAX_PROCESS];
int front;
int rear;
int size;
};
void initQueue(struct ProcessQueue* q) {
q->front = 0;
q->rear = 0;
q->size = 0;
}
int isQueueEmpty(struct ProcessQueue* q) {
return q->size == 0;
}
int isQueueFull(struct ProcessQueue* q) {
return q->size == MAX_PROCESS;
}
void enqueue(struct ProcessQueue* q, struct Process* p) {
if (isQueueFull(q)) {
printf("Queue is full. Cannot enqueue process %d.\n", p->id);
return;
}
q->queue[q->rear] = p;
q->rear = (q->rear + 1) % MAX_PROCESS;
q->size++;
}
struct Process* dequeue(struct ProcessQueue* q) {
if (isQueueEmpty(q)) {
printf("Queue is empty. Cannot dequeue process.\n");
return NULL;
}
struct Process* p = q->queue[q->front];
q->front = (q->front + 1) % MAX_PROCESS;
q->size--;
return p;
}
void printQueue(struct ProcessQueue* q) {
if (isQueueEmpty(q)) {
printf("Queue is empty.\n");
return;
}
printf("Queue:\n");
int i;
for (i = q->front; i != q->rear; i = (i + 1) % MAX_PROCESS) {
printf(" Process %d (arrival time: %d, burst time: %d, priority: %d)\n", q->queue[i]->id, q->queue[i]->arrival_time, q->queue[i]->burst_time, q->queue[i]->priority);
}
}
int main() {
int n;
printf("Enter the number of processes: ");
scanf("%d", &n);
if (n > MAX_PROCESS) {
printf("Number of processes cannot exceed %d.\n", MAX_PROCESS);
return 1;
}
struct Process processes[MAX_PROCESS];
int i;
for (i = 0; i < n; i++) {
printf("Enter arrival time, burst time, and priority for process %d: ", i);
struct Process* p = (struct Process*)malloc(sizeof(struct Process));
p->id = i;
scanf("%d %d %d", &p->arrival_time, &p->burst_time, &p->priority);
processes[i] = *p;
}
struct ProcessQueue queue;
initQueue(&queue);
int time = 0;
while (!isQueueEmpty(&queue) || time == 0) {
for (i = 0; i < n; i++) {
if (processes[i].arrival_time == time) {
enqueue(&queue, &processes[i]);
}
}
if (!isQueueEmpty(&queue)) {
struct Process* p = dequeue(&queue);
printf("Process %d is running (arrival time: %d, burst time: %d, priority: %d) at time %d.\n", p->id, p->arrival_time, p->burst_time, p->priority, time);
p->burst_time--;
if (p->burst_time == 0) {
printf("Process %d is finished at time %d.\n", p->id, time + 1);
} else {
enqueue(&queue, p);
}
}
time++;
}
return 0;
}
```
该示例代码需要用户输入进程数量以及每个进程的到达时间、执行时间和优先级等信息。程序首先将所有进程按照到达时间插入到一个等待队列中,然后按照优先级从高到低依次执行队列中的进程。如果有多个进程的优先级相同,则按照先到达的顺序依次执行。在每个时间片中,程序会检查是否有新的进程到达,以及当前正在执行的进程是否已经完成。如果正在执行的进程完成了,那么程序会将其从队列中移除,否则程序会将其重新加入队列中等待下一次执行。最后,程序将输出每个进程的完成时间。
需要注意的是,该示例代码并没有考虑一些实际情况,例如进程的等待时间、响应时间和周转时间等指标,用户可以根据需要自行添加。
相关推荐
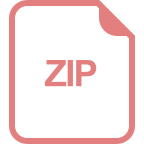
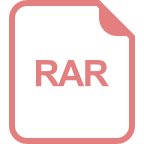












