四种进程调度算法模拟实现生成c语言代码能够运行
时间: 2023-12-18 19:04:06 浏览: 41
好的,我可以为您提供四种进程调度算法模拟实现的C语言代码,包括先来先服务(FCFS)、短作业优先(SJF)、优先级调度和时间片轮转调度。
1. 先来先服务(FCFS)算法:
```c
#include <stdio.h>
#include <stdlib.h>
struct pcb {
int pid; // 进程ID
int burst_time; // 进程执行时间
int arrival_time; // 进程到达时间
int waiting_time; // 进程等待时间
int turnaround_time; // 进程周转时间
};
void fcfs(struct pcb *processes, int n) {
int current_time = 0;
int total_waiting_time = 0;
int total_turnaround_time = 0;
printf("进程ID\t到达时间\t执行时间\t等待时间\t周转时间\n");
for (int i = 0; i < n; i++) {
struct pcb process = processes[i];
process.waiting_time = current_time - process.arrival_time;
current_time += process.burst_time;
process.turnaround_time = current_time - process.arrival_time;
total_waiting_time += process.waiting_time;
total_turnaround_time += process.turnaround_time;
printf("%d\t%d\t\t%d\t\t%d\t\t%d\n", process.pid, process.arrival_time, process.burst_time, process.waiting_time, process.turnaround_time);
}
printf("平均等待时间:%f\n", (float)total_waiting_time / n);
printf("平均周转时间:%f\n", (float)total_turnaround_time / n);
}
int main() {
struct pcb processes[] = {
{1, 5, 0, 0, 0},
{2, 3, 1, 0, 0},
{3, 2, 2, 0, 0},
{4, 1, 4, 0, 0},
{5, 4, 5, 0, 0}
};
int n = sizeof(processes) / sizeof(processes[0]);
fcfs(processes, n);
return 0;
}
```
2. 短作业优先(SJF)算法:
```c
#include <stdio.h>
#include <stdlib.h>
struct pcb {
int pid; // 进程ID
int burst_time; // 进程执行时间
int arrival_time; // 进程到达时间
int waiting_time; // 进程等待时间
int turnaround_time; // 进程周转时间
};
void sjf(struct pcb *processes, int n) {
int current_time = 0;
int total_waiting_time = 0;
int total_turnaround_time = 0;
int completed = 0;
int *burst_times = (int *)malloc(sizeof(int) * n);
for (int i = 0; i < n; i++) {
burst_times[i] = processes[i].burst_time;
}
printf("进程ID\t到达时间\t执行时间\t等待时间\t周转时间\n");
while (completed != n) {
int shortest_job = -1;
int shortest_burst_time = 999;
for (int i = 0; i < n; i++) {
if (processes[i].arrival_time <= current_time && burst_times[i] < shortest_burst_time && burst_times[i] > 0) {
shortest_job = i;
shortest_burst_time = burst_times[i];
}
}
if (shortest_job == -1) {
current_time++;
} else {
struct pcb process = processes[shortest_job];
process.waiting_time = current_time - process.arrival_time;
current_time += process.burst_time;
process.turnaround_time = current_time - process.arrival_time;
total_waiting_time += process.waiting_time;
total_turnaround_time += process.turnaround_time;
processes[shortest_job] = process;
burst_times[shortest_job] = 0;
completed++;
printf("%d\t%d\t\t%d\t\t%d\t\t%d\n", process.pid, process.arrival_time, process.burst_time, process.waiting_time, process.turnaround_time);
}
}
printf("平均等待时间:%f\n", (float)total_waiting_time / n);
printf("平均周转时间:%f\n", (float)total_turnaround_time / n);
}
int main() {
struct pcb processes[] = {
{1, 5, 0, 0, 0},
{2, 3, 1, 0, 0},
{3, 2, 2, 0, 0},
{4, 1, 4, 0, 0},
{5, 4, 5, 0, 0}
};
int n = sizeof(processes) / sizeof(processes[0]);
sjf(processes, n);
return 0;
}
```
3. 优先级调度算法:
```c
#include <stdio.h>
#include <stdlib.h>
struct pcb {
int pid; // 进程ID
int burst_time; // 进程执行时间
int arrival_time; // 进程到达时间
int priority; // 进程优先级
int waiting_time; // 进程等待时间
int turnaround_time; // 进程周转时间
};
void priority(struct pcb *processes, int n) {
int current_time = 0;
int total_waiting_time = 0;
int total_turnaround_time = 0;
int completed = 0;
printf("进程ID\t到达时间\t执行时间\t优先级\t等待时间\t周转时间\n");
while (completed != n) {
int highest_priority_job = -1;
int highest_priority = -1;
for (int i = 0; i < n; i++) {
if (processes[i].arrival_time <= current_time && processes[i].priority > highest_priority && processes[i].burst_time > 0) {
highest_priority_job = i;
highest_priority = processes[i].priority;
}
}
if (highest_priority_job == -1) {
current_time++;
} else {
struct pcb process = processes[highest_priority_job];
process.waiting_time = current_time - process.arrival_time;
current_time += process.burst_time;
process.turnaround_time = current_time - process.arrival_time;
total_waiting_time += process.waiting_time;
total_turnaround_time += process.turnaround_time;
processes[highest_priority_job] = process;
completed++;
printf("%d\t%d\t\t%d\t\t%d\t\t%d\t\t%d\n", process.pid, process.arrival_time, process.burst_time, process.priority, process.waiting_time, process.turnaround_time);
}
}
printf("平均等待时间:%f\n", (float)total_waiting_time / n);
printf("平均周转时间:%f\n", (float)total_turnaround_time / n);
}
int main() {
struct pcb processes[] = {
{1, 5, 0, 3, 0, 0},
{2, 3, 1, 2, 0, 0},
{3, 2, 2, 4, 0, 0},
{4, 1, 4, 1, 0, 0},
{5, 4, 5, 5, 0, 0}
};
int n = sizeof(processes) / sizeof(processes[0]);
priority(processes, n);
return 0;
}
```
4. 时间片轮转调度算法:
```c
#include <stdio.h>
#include <stdlib.h>
struct pcb {
int pid; // 进程ID
int burst_time; // 进程执行时间
int arrival_time; // 进程到达时间
int remaining_time; // 进程剩余执行时间
int waiting_time; // 进程等待时间
int turnaround_time; // 进程周转时间
};
void round_robin(struct pcb *processes, int n, int quantum) {
int current_time = 0;
int total_waiting_time = 0;
int total_turnaround_time = 0;
int completed = 0;
printf("进程ID\t到达时间\t执行时间\t等待时间\t周转时间\n");
while (completed != n) {
for (int i = 0; i < n; i++) {
if (processes[i].arrival_time <= current_time && processes[i].remaining_time > 0) {
if (processes[i].remaining_time <= quantum) {
current_time += processes[i].remaining_time;
processes[i].remaining_time = 0;
processes[i].waiting_time = current_time - processes[i].arrival_time - processes[i].burst_time;
processes[i].turnaround_time = current_time - processes[i].arrival_time;
total_waiting_time += processes[i].waiting_time;
total_turnaround_time += processes[i].turnaround_time;
completed++;
printf("%d\t%d\t\t%d\t\t%d\t\t%d\n", processes[i].pid, processes[i].arrival_time, processes[i].burst_time, processes[i].waiting_time, processes[i].turnaround_time);
} else {
current_time += quantum;
processes[i].remaining_time -= quantum;
}
}
}
}
printf("平均等待时间:%f\n", (float)total_waiting_time / n);
printf("平均周转时间:%f\n", (float)total_turnaround_time / n);
}
int main() {
struct pcb processes[] = {
{1, 10, 0, 10, 0, 0},
{2, 5, 1, 5, 0, 0},
{3, 8, 2, 8, 0, 0},
{4, 7, 3, 7, 0, 0},
{5, 6, 4, 6, 0, 0}
};
int n = sizeof(processes) / sizeof(processes[0]);
int quantum = 2;
round_robin(processes, n, quantum);
return 0;
}
```
以上是四种进程调度算法模拟实现的C语言代码,您可以根据需要进行修改和优化。
相关推荐
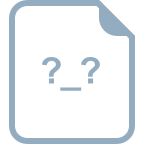
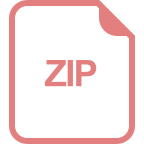
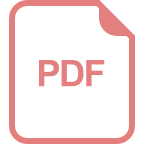














