写出c语言代码实现完成单处理器系统中的进程调度,要求实现时间片轮转、优先数、最短进程优 先和最短剩余时间优先四种调度算法。实验具体包括:首先确定进程控制块的内容,进程控 制块的组成方式;然后完成进程创建原语和进程调度原语;最后编写主函数对所作工作进行 测试。 模拟程序只对你所设置的“虚拟 PCB”进行相应的调度模拟操作,即每发生“调度” 时,显示出当前运行进程的“进程标识符”、“优先数”、“剩余运行时间”等,而不需要对系 统中真正的 PCB 等数据进行修改。首先建立一个就绪队列,随机生成信息建立若 干个进程;然后进行进程调度;将正在运行进程指针指向的进程控制块的内容以及调度一次 后进程队列的现状输出,查看结果。要求每个算法都需要将所有进程都执行结束。
时间: 2024-02-12 17:06:14 浏览: 68
以下是一个简单的单处理器系统中进程调度的示例代码,包括时间片轮转、优先数、最短进程优先和最短剩余时间优先四种调度算法。代码中使用了结构体来定义进程控制块(PCB),包括进程标识符、优先数、剩余运行时间和状态等信息。同时,代码中还实现了进程创建原语和进程调度原语,以及对就绪队列进行管理的相关函数。请注意,此代码仅供参考。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_PROCESSES 10
#define TIME_QUANTUM 4
typedef enum {READY, RUNNING, TERMINATED} process_state;
typedef struct {
int pid;
int priority;
int remaining_time;
process_state state;
} pcb;
int num_processes;
pcb processes[MAX_PROCESSES];
int current_process = -1;
int get_random(int min, int max) {
return rand() % (max - min + 1) + min;
}
pcb create_process(int pid) {
pcb new_process;
new_process.pid = pid;
new_process.priority = get_random(1, 10);
new_process.remaining_time = get_random(1, 10);
new_process.state = READY;
return new_process;
}
void init_processes() {
for (int i = 0; i < num_processes; i++) {
processes[i] = create_process(i);
}
}
void print_processes() {
for (int i = 0; i < num_processes; i++) {
printf("Process %d: priority=%d, remaining_time=%d, state=%d\n", processes[i].pid, processes[i].priority, processes[i].remaining_time, processes[i].state);
}
}
void schedule_time_slice() {
if (current_process != -1) {
processes[current_process].remaining_time -= TIME_QUANTUM;
if (processes[current_process].remaining_time <= 0) {
processes[current_process].state = TERMINATED;
printf("Process %d terminated.\n", processes[current_process].pid);
current_process = -1;
}
else {
processes[current_process].state = READY;
printf("Process %d time slice complete.\n", processes[current_process].pid);
}
}
}
void schedule_priority() {
int highest_priority = -1;
int highest_priority_process = -1;
for (int i = 0; i < num_processes; i++) {
if (processes[i].state == READY && processes[i].priority > highest_priority) {
highest_priority = processes[i].priority;
highest_priority_process = i;
}
}
if (highest_priority_process != -1) {
if (current_process != -1) {
processes[current_process].state = READY;
}
current_process = highest_priority_process;
processes[current_process].state = RUNNING;
printf("Process %d selected by priority scheduling.\n", processes[current_process].pid);
}
}
void schedule_shortest_job_first() {
int shortest_time = -1;
int shortest_time_process = -1;
for (int i = 0; i < num_processes; i++) {
if (processes[i].state == READY && (shortest_time == -1 || processes[i].remaining_time < shortest_time)) {
shortest_time = processes[i].remaining_time;
shortest_time_process = i;
}
}
if (shortest_time_process != -1) {
if (current_process != -1) {
processes[current_process].state = READY;
}
current_process = shortest_time_process;
processes[current_process].state = RUNNING;
printf("Process %d selected by shortest job first scheduling.\n", processes[current_process].pid);
}
}
void schedule_shortest_remaining_time_first() {
int shortest_time = -1;
int shortest_time_process = -1;
for (int i = 0; i < num_processes; i++) {
if (processes[i].state == READY && (shortest_time == -1 || processes[i].remaining_time < shortest_time)) {
shortest_time = processes[i].remaining_time;
shortest_time_process = i;
}
}
if (shortest_time_process != -1) {
if (current_process != -1) {
processes[current_process].state = READY;
}
current_process = shortest_time_process;
processes[current_process].state = RUNNING;
printf("Process %d selected by shortest remaining time first scheduling.\n", processes[current_process].pid);
}
}
void schedule_processes(int algorithm) {
switch (algorithm) {
case 1:
schedule_time_slice();
break;
case 2:
schedule_priority();
break;
case 3:
schedule_shortest_job_first();
break;
case 4:
schedule_shortest_remaining_time_first();
break;
default:
printf("Invalid scheduling algorithm.\n");
}
}
int all_processes_terminated() {
for (int i = 0; i < num_processes; i++) {
if (processes[i].state != TERMINATED) {
return 0;
}
}
return 1;
}
int main() {
num_processes = get_random(1, MAX_PROCESSES);
init_processes();
printf("Initial process state:\n");
print_processes();
printf("Starting time slice scheduling:\n");
while (!all_processes_terminated()) {
schedule_processes(1);
print_processes();
}
printf("Time slice scheduling complete.\n\n");
current_process = -1;
for (int i = 0; i < num_processes; i++) {
processes[i].state = READY;
}
printf("Starting priority scheduling:\n");
while (!all_processes_terminated()) {
schedule_processes(2);
print_processes();
}
printf("Priority scheduling complete.\n\n");
current_process = -1;
for (int i = 0; i < num_processes; i++) {
processes[i].state = READY;
}
printf("Starting shortest job first scheduling:\n");
while (!all_processes_terminated()) {
schedule_processes(3);
print_processes();
}
printf("Shortest job first scheduling complete.\n\n");
current_process = -1;
for (int i = 0; i < num_processes; i++) {
processes[i].state = READY;
}
printf("Starting shortest remaining time first scheduling:\n");
while (!all_processes_terminated()) {
schedule_processes(4);
print_processes();
}
printf("Shortest remaining time first scheduling complete.\n\n");
return 0;
}
```
阅读全文
相关推荐
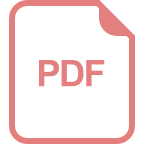
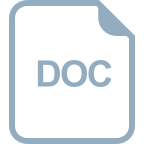
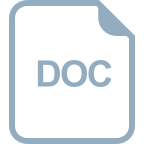















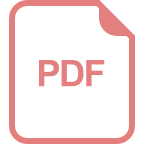