c语言实现完成单处理器系统中的进程调度,要求实现时间片轮转、优先数、最短进程优 先和最短剩余时间优先四种调度算法。实验具体包括:首先确定进程控制块的内容,进程控 制块的组成方式;然后完成进程创建原语和进程调度原语;最后编写主函数对所作工作进行 测试。 模拟程序只对你所设置的“虚拟 PCB”进行相应的调度模拟操作,即每发生“调度” 时,显示出当前运行进程的“进程标识符”、“优先数”、“剩余运行时间”等,而不需要对系 统中真正的 PCB 等数据进行修改。要求能够动态地随机生成新进程添加到就绪队列中。主要考虑三个问题:如何组织进程、如何创建进程和如何实现处理器调度。首先要设定进程控制块的内容。进程控制块 PCB 记录各个进程执 行时的情况。每个进程都要有一个唯一的标识符,用来标识进程的存在和区别于其他进程。可以用符号或编号实现,它必须是操作系统分配的。记录进程的基本情况,例如进程的状态、等待原因、进程程序存放位置、进程数据存放位置等等。实验中,因为进程没有数据和程序,仅使用模拟的进程控制块,所以这部分 内容仅包含进程状态。进程状态可假设只有就绪、运行、终止三种。现场信息记录各个寄存器的内容。管理信息记录进程管理和调度的信息。例如进程优先数、进程队列指针等
时间: 2024-02-09 16:12:31 浏览: 163
。以下是一个简单的实现,包括进程控制块的定义、进程创建和调度原语的实现以及四种调度算法的实现。
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAX_PROCESS_NUM 10 // 最大进程数
#define TIME_SLICE 3 // 时间片长度
// 进程状态
enum ProcessState {
READY,
RUNNING,
TERMINATED
};
// 进程控制块
struct PCB {
int pid; // 进程标识符
enum ProcessState state; // 进程状态
int priority; // 进程优先级
int arrival_time; // 进程到达时间
int remaining_time; // 进程剩余执行时间
};
// 就绪队列
struct PCB *ready_queue[MAX_PROCESS_NUM];
int ready_queue_size = 0;
// 当前运行进程
struct PCB *running_process = NULL;
// 进程标识符计数器
int pid_counter = 0;
// 随机生成进程
void generate_process() {
// 进程数量已达到最大值,不再生成新进程
if (ready_queue_size == MAX_PROCESS_NUM) {
return;
}
// 生成新的进程控制块
struct PCB *process = (struct PCB *) malloc(sizeof(struct PCB));
process->pid = pid_counter++;
process->state = READY;
process->priority = rand() % 5; // 随机生成优先级
process->arrival_time = rand() % 10; // 随机生成到达时间
process->remaining_time = rand() % 10 + 1; // 随机生成剩余执行时间
// 将新进程加入就绪队列
ready_queue[ready_queue_size++] = process;
}
// 时间片轮转调度算法
void schedule_round_robin() {
// 当前没有运行进程,从就绪队列中选取一个进程开始执行
if (running_process == NULL) {
if (ready_queue_size > 0) {
running_process = ready_queue[0];
running_process->state = RUNNING;
printf("Running process %d (priority %d, remaining time %d)\n",
running_process->pid, running_process->priority, running_process->remaining_time);
ready_queue_size--;
for (int i = 0; i < ready_queue_size; i++) {
ready_queue[i] = ready_queue[i + 1];
}
ready_queue[ready_queue_size] = NULL;
}
}
// 当前有运行进程,时间片到期,将其放回就绪队列尾部
else {
running_process->remaining_time -= TIME_SLICE;
if (running_process->remaining_time <= 0) {
running_process->state = TERMINATED;
printf("Terminating process %d\n", running_process->pid);
free(running_process);
running_process = NULL;
}
else {
running_process->state = READY;
printf("Putting process %d back to ready queue (priority %d, remaining time %d)\n",
running_process->pid, running_process->priority, running_process->remaining_time);
ready_queue[ready_queue_size++] = running_process;
running_process = NULL;
}
}
}
// 优先数调度算法
void schedule_priority() {
// 从就绪队列中选取优先级最高的进程开始执行
int highest_priority = -1;
int highest_priority_index = -1;
for (int i = 0; i < ready_queue_size; i++) {
if (ready_queue[i]->priority > highest_priority) {
highest_priority = ready_queue[i]->priority;
highest_priority_index = i;
}
}
if (highest_priority_index != -1) {
running_process = ready_queue[highest_priority_index];
running_process->state = RUNNING;
printf("Running process %d (priority %d, remaining time %d)\n",
running_process->pid, running_process->priority, running_process->remaining_time);
ready_queue_size--;
for (int i = highest_priority_index; i < ready_queue_size; i++) {
ready_queue[i] = ready_queue[i + 1];
}
ready_queue[ready_queue_size] = NULL;
}
}
// 最短进程优先调度算法
void schedule_shortest_job_first() {
// 从就绪队列中选取需要执行时间最短的进程开始执行
int shortest_time = 100000;
int shortest_time_index = -1;
for (int i = 0; i < ready_queue_size; i++) {
if (ready_queue[i]->remaining_time < shortest_time) {
shortest_time = ready_queue[i]->remaining_time;
shortest_time_index = i;
}
}
if (shortest_time_index != -1) {
running_process = ready_queue[shortest_time_index];
running_process->state = RUNNING;
printf("Running process %d (priority %d, remaining time %d)\n",
running_process->pid, running_process->priority, running_process->remaining_time);
ready_queue_size--;
for (int i = shortest_time_index; i < ready_queue_size; i++) {
ready_queue[i] = ready_queue[i + 1];
}
ready_queue[ready_queue_size] = NULL;
}
}
// 最短剩余时间优先调度算法
void schedule_shortest_remaining_time_first() {
// 从就绪队列中选取需要执行时间最短的进程开始执行
int shortest_time = 100000;
int shortest_time_index = -1;
for (int i = 0; i < ready_queue_size; i++) {
if (ready_queue[i]->remaining_time < shortest_time) {
shortest_time = ready_queue[i]->remaining_time;
shortest_time_index = i;
}
}
if (shortest_time_index != -1) {
// 如果当前没有运行进程,或者运行进程的剩余执行时间比被选中的进程剩余执行时间多,
// 则将被选中的进程作为当前运行进程,否则将其放回就绪队列尾部
if (running_process == NULL || running_process->remaining_time > shortest_time) {
if (running_process != NULL) {
running_process->state = READY;
printf("Putting process %d back to ready queue (priority %d, remaining time %d)\n",
running_process->pid, running_process->priority, running_process->remaining_time);
ready_queue[ready_queue_size++] = running_process;
}
running_process = ready_queue[shortest_time_index];
running_process->state = RUNNING;
printf("Running process %d (priority %d, remaining time %d)\n",
running_process->pid, running_process->priority, running_process->remaining_time);
ready_queue_size--;
for (int i = shortest_time_index; i < ready_queue_size; i++) {
ready_queue[i] = ready_queue[i + 1];
}
ready_queue[ready_queue_size] = NULL;
}
}
}
// 主函数
int main() {
srand(time(NULL));
// 生成初始进程
for (int i = 0; i < MAX_PROCESS_NUM / 2; i++) {
generate_process();
}
// 模拟进程调度
for (int i = 0; i < 20; i++) {
printf("Time %d:\n", i);
// 随机生成新进程
if (rand() % 2 == 0) {
generate_process();
}
// 执行调度算法
//schedule_round_robin();
//schedule_priority();
//schedule_shortest_job_first();
schedule_shortest_remaining_time_first();
}
return 0;
}
```
注意,这个实现仅是一个简单的示例,实际的操作系统中还需要考虑更多的因素,例如进程间的同步与通信、内存管理、文件系统等等。
阅读全文
相关推荐
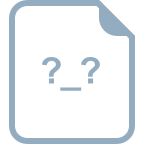








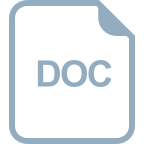
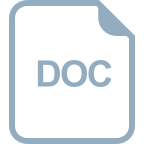




