C++Design a sorting function template, and use integer, floating point and string sorting in the main function to verify. int main(){ string Str[5]={"Zhang","Li","Wang","Qian","Liang"}; int Int[4]={20,12,0,-5}; double Dou[6]={11,3,5,6,10,-9}; Sort<string>(Str,5); Sort<int>(Int,4); Sort<double>(Dou,6); Print(Str,5); Print(Int,4); Print(Dou,6); return 0; }
时间: 2024-02-18 19:04:46 浏览: 27
Sure, here's an example of a sorting function template that can be used to sort arrays of different data types:
```c++
#include <iostream>
#include <string>
template <typename T>
void Sort(T arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (arr[i] > arr[j]) {
T temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
}
template <typename T>
void Print(T arr[], int n) {
for (int i = 0; i < n; i++) {
std::cout << arr[i] << " ";
}
std::cout << std::endl;
}
int main(){
std::string Str[5]={"Zhang","Li","Wang","Qian","Liang"};
int Int[4]={20,12,0,-5};
double Dou[6]={11,3,5,6,10,-9};
Sort<std::string>(Str,5);
Sort<int>(Int,4);
Sort<double>(Dou,6);
Print<std::string>(Str,5);
Print<int>(Int,4);
Print<double>(Dou,6);
return 0;
}
```
In this example, we have two function templates: `Sort` and `Print`. The `Sort` template takes an array of type `T` and its size, and sorts the array in ascending order using a simple bubble sort algorithm. The `Print` template takes an array of type `T` and its size, and prints the elements of the array to the console.
In the `main` function, we create three arrays of different types (string, integer, and double), fill them with some values, sort them using the `Sort` function template, and then print them using the `Print` function template.
You can compile and run this program to see the sorting in action. The output should look something like this:
```
Liang Li Qian Wang Zhang
-5 0 12 20
-9 3 5 6 10 11
```
相关推荐
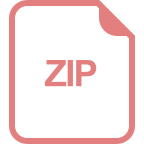















