上文代码加上 public TreeNode createTree(String str) { Stack<TreeNode> st=new Stack<TreeNode>(); TreeNode p=null; boolean flag=true; char ch; int i=0; while (i<str.length()) ch=str.charAt(i); switch(ch) { case '(': st.push(p); flag=true; break; case ')': st.pop(); break; case ',': flag=false; break; default: p=new TreeNode(ch); if (root==null) root=p; else { if (flag) { if (!st.empty()) st.peek().leftChild=p; } else { if (!st.empty()) st.peek().rightChild=p; } } break; } i++; } return root; } public static class TreeNode { private TreeNode leftChild; private TreeNode rightChild; private char item; public TreeNode(char item) { this(null, null, item); } public TreeNode(TreeNode leftChild, TreeNode rightChild, char item) { this.leftChild = leftChild; this.rightChild = rightChild; this.item = item; } } }
时间: 2024-03-14 16:48:36 浏览: 89
好的,那么我来补全代码。请注意,下面的代码可能需要一些微调才能完全适应您的程序。
```java
public class Main6 {
private TreeNode root;
public Main6() {
root = null;
}
public TreeNode createTree(String str) {
Stack<TreeNode> st = new Stack<TreeNode>();
TreeNode p = null;
boolean flag = true;
char ch;
int i = 0;
while (i < str.length()) {
ch = str.charAt(i);
switch(ch) {
case '(':
st.push(p);
flag = true;
break;
case ')':
st.pop();
break;
case ',':
flag = false;
break;
default:
p = new TreeNode(ch);
if (root == null) {
root = p;
} else {
if (flag) {
if (!st.empty()) {
st.peek().leftChild = p;
}
} else {
if (!st.empty()) {
st.peek().rightChild = p;
}
}
}
break;
}
i++;
}
return root;
}
public void inOrder(TreeNode root) {
if (root == null) {
return;
}
inOrder(root.leftChild);
System.out.print(root.item + " ");
inOrder(root.rightChild);
}
public static class TreeNode {
private TreeNode leftChild;
private TreeNode rightChild;
private char item;
public TreeNode(char item) {
this(null, null, item);
}
public TreeNode(TreeNode leftChild, TreeNode rightChild, char item) {
this.leftChild = leftChild;
this.rightChild = rightChild;
this.item = item;
}
}
}
```
需要注意的是,这段代码中的 `TreeNode` 类需要有 `leftChild` 和 `rightChild` 两个属性,表示左子节点和右子节点。另外,程序中的 `root` 变量需要在类中定义。
阅读全文
相关推荐
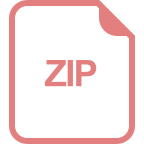
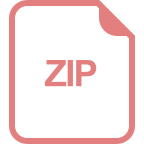
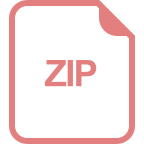














