用非递归实现二叉树的前序、中序、后序遍历算法C语言完整程序代码
时间: 2023-07-10 08:10:49 浏览: 40
好的,下面是用C语言实现的完整程序代码,包括二叉树的定义和遍历算法的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <stack.h>
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 创建二叉树
TreeNode* createTree() {
int val;
scanf("%d", &val);
if (val == -1) {
return NULL;
}
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = val;
root->left = createTree();
root->right = createTree();
return root;
}
// 前序遍历
void preorder(TreeNode* root) {
if (root == NULL) {
return;
}
stack<TreeNode*> s;
s.push(root);
while (!s.empty()) {
TreeNode* node = s.top();
s.pop();
printf("%d ", node->val); // 访问节点
if (node->right != NULL) { // 先将右子节点入栈
s.push(node->right);
}
if (node->left != NULL) { // 再将左子节点入栈
s.push(node->left);
}
}
}
// 中序遍历
void inorder(TreeNode* root) {
if (root == NULL) {
return;
}
stack<TreeNode*> s;
TreeNode* node = root;
while (node != NULL || !s.empty()) {
if (node != NULL) { // 如果当前节点不为空,将其压入栈中,并将当前节点更新为其左子节点
s.push(node);
node = node->left;
} else { // 如果当前节点为空,弹出栈顶元素,访问该节点,并将当前节点更新为其右子节点
node = s.top();
s.pop();
printf("%d ", node->val); // 访问节点
node = node->right;
}
}
}
// 后序遍历
void postorder(TreeNode* root) {
if (root == NULL) {
return;
}
stack<TreeNode*> s1, s2;
s1.push(root);
while (!s1.empty()) {
TreeNode* node = s1.top();
s1.pop();
s2.push(node);
if (node->left != NULL) { // 先将左子节点入栈
s1.push(node->left);
}
if (node->right != NULL) { // 再将右子节点入栈
s1.push(node->right);
}
}
while (!s2.empty()) {
TreeNode* node = s2.top();
s2.pop();
printf("%d ", node->val); // 访问节点
}
}
int main() {
TreeNode* root = createTree();
printf("前序遍历:");
preorder(root);
printf("\n中序遍历:");
inorder(root);
printf("\n后序遍历:");
postorder(root);
printf("\n");
return 0;
}
```
以上就是用C语言实现的完整程序代码。
相关推荐
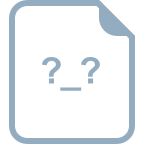














