hashmap for 遍历原理
时间: 2024-02-01 12:10:37 浏览: 26
HashMap的遍历原理如下:
1. 首先,HashMap会将所有的键值对存储在一个数组中,这个数组被称为哈希桶(hash bucket)。
2. 当我们向HashMap中插入一个键值对时,HashMap会根据键的哈希码计算出一个索引值,然后将键值对存储在对应的索引位置上。
3. 当我们要遍历HashMap时,可以通过遍历哈希桶来获取所有的键值对。
4. 遍历哈希桶的方式有两种:迭代器(Iterator)和增强for循环(foreach)。
- 使用迭代器遍历HashMap时,首先通过调用HashMap的entrySet()方法获取到一个包含所有键值对的Set集合,然后通过调用Set集合的iterator()方法获取到一个迭代器,最后使用迭代器的next()方法和hasNext()方法来遍历键值对。
- 使用增强for循环遍历HashMap时,直接使用HashMap的entrySet()方法获取到一个包含所有键值对的Set集合,然后使用增强for循环来遍历键值对。
下面是使用迭代器和增强for循环遍历HashMap的示例代码:
```java
import java.util.HashMap;import java.util.Iterator;
import java.util.Map;
public class HashMapTraversalExample {
public static void main(String[] args) {
// 创建一个HashMap
HashMap<String, Integer> hashMap = new HashMap<>();
hashMap.put("apple", 1);
hashMap.put("banana", 2);
hashMap.put("orange", 3);
// 使用迭代器遍历HashMap
Iterator<Map.Entry<String, Integer>> iterator = hashMap.entrySet().iterator();
while (iterator.hasNext()) {
Map.Entry<String, Integer> entry = iterator.next();
String key = entry.getKey();
Integer value = entry.getValue();
System.out.println("Key: " + key + ", Value: " + value);
}
// 使用增强for循环遍历HashMap
for (Map.Entry<String, Integer> entry : hashMap.entrySet()) {
String key = entry.getKey();
Integer value = entry.getValue();
System.out.println("Key: " + key + ", Value: " + value);
}
}
}
```
相关推荐
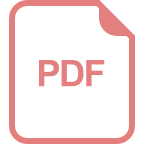














