public int RetBooks(Borrow r) { int ret = 0; try{ borCon.connectDB(); String sqlret = "update borrow set state='"+"0"+"' where ISBN='" + r.getIsbn() + "' and readerID=" + r.getID(); borCon.statement = borCon.connection.prepareStatement(sqlret); ret = borCon.statement.executeUpdate(sqlret); } catch (SQLException throwable) { throwable.printStackTrace(); }finally { borCon.close(); } return ret; }
时间: 2024-02-14 07:20:57 浏览: 25
这段代码看起来是一个 Java 方法,名为 RetBooks,输入参数为一个 Borrow 对象,输出为一个整型值。它的作用是更新 borrow 表中的某一行数据,将其 state 字段的值设为 0,这个更新操作是根据输入的 Borrow 对象中的 ISBN 和 readerID 字段所对应的值来进行的。在方法中,首先建立了一个数据库连接,然后构造了一个 SQL 更新语句,接着使用 PreparedStatement 对象执行这个 SQL 语句,并返回执行结果。如果在执行过程中发生了异常,会将异常信息打印出来,最后无论是否发生异常都会关闭数据库连接。
相关问题
//图书借出+1 public int BorrowAdd(Borrow r) { int ret = 0; try{ borCon.connectDB(); String sql1 = "select borrow from books where ISBN='" + r.getIsbn() + "'"; borCon.statement = borCon.connection.prepareStatement(sql1); borCon.resultSet = borCon.statement.executeQuery(sql1); int borrow = -1; if(borCon.resultSet .next()){ borrow = borCon.resultSet .getInt("borrow") + 1; } String sql2 = "update books set borrow='" + borrow + "' where ISBN='" + r.getIsbn() + "';"; borCon.statement = borCon.connection.prepareStatement(sql2); ret = borCon.statement.executeUpdate(sql2); } catch (SQLException throwable) { throwable.printStackTrace(); }finally { borCon.close(); } return ret; }翻译这段代码
这段代码定义了一个名为BorrowAdd的方法,其参数为一个Borrow对象,返回一个整数类型的值。该方法的作用是连接到数据库,首先执行查询语句以获取指定ISBN编号图书的借出数量,然后将其加1,最后再执行更新语句将借出数量更新到数据库中。如果在查询或更新过程中发生异常,则将其打印出来。无论是否发生异常,最终都会关闭连接。最后,该方法返回执行更新语句所影响的记录数。
public class BorrowSQL { ConnectionSQL borCon = new ConnectionSQL(); //判断是否超出借阅数量限制 public int check(Borrow r) { int borrowing = -1; try{ borCon.connectDB(); String sql = "select COUNT(readerID) from borrow where readerID=" + r.getID(); borCon.statement = borCon.connection.prepareStatement(sql); borCon.resultSet = borCon.statement.executeQuery(sql); if(borCon.resultSet .next()){ borrowing = borCon.resultSet .getInt("COUNT(readerID)"); } } catch (SQLException throwable) { throwable.printStackTrace(); }finally { borCon.close(); } return borrowing; }翻译一下这段代码
这段代码定义了一个名为BorrowSQL的类,其中包含一个名为borCon的ConnectionSQL对象。该类还有一个名为check的方法,其参数为一个Borrow对象,返回一个整数类型的值。该方法的作用是连接到数据库,执行查询语句以获取指定读者已借阅的数量,然后将其返回。如果在查询过程中发生异常,则将其打印出来。无论是否发生异常,最终都会关闭连接。
相关推荐
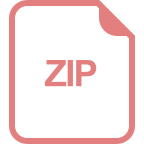













