使用c语言编写图书管理系统
时间: 2023-12-11 12:54:29 浏览: 61
好的,下面是一个简单的图书管理系统的 C 语言代码,仅供参考:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义图书结构体
struct Book {
int id;
char name[50];
char author[50];
char publisher[50];
double price;
};
// 定义函数:展示菜单
void showMenu() {
printf("=============================\n");
printf("图书管理系统\n");
printf("1. 添加图书\n");
printf("2. 删除图书\n");
printf("3. 修改图书信息\n");
printf("4. 查询图书\n");
printf("0. 退出系统\n");
printf("=============================\n");
}
// 定义函数:添加图书
void addBook(struct Book books[], int *count) {
printf("请输入图书信息:\n");
printf("编号:");
scanf("%d", &books[*count].id);
printf("书名:");
scanf("%s", books[*count].name);
printf("作者:");
scanf("%s", books[*count].author);
printf("出版社:");
scanf("%s", books[*count].publisher);
printf("价格:");
scanf("%lf", &books[*count].price);
(*count)++;
printf("添加成功!\n");
}
// 定义函数:删除图书
void deleteBook(struct Book books[], int *count) {
int id, index = -1;
printf("请输入待删除图书编号:");
scanf("%d", &id);
for (int i = 0; i < *count; i++) {
if (books[i].id == id) {
index = i;
break;
}
}
if (index == -1) {
printf("未找到该图书!\n");
} else {
for (int i = index; i < *count - 1; i++) {
books[i] = books[i + 1];
}
(*count)--;
printf("删除成功!\n");
}
}
// 定义函数:修改图书信息
void modifyBook(struct Book books[], int count) {
int id, index = -1;
printf("请输入待修改图书编号:");
scanf("%d", &id);
for (int i = 0; i < count; i++) {
if (books[i].id == id) {
index = i;
break;
}
}
if (index == -1) {
printf("未找到该图书!\n");
} else {
printf("请输入图书信息:\n");
printf("书名:");
scanf("%s", books[index].name);
printf("作者:");
scanf("%s", books[index].author);
printf("出版社:");
scanf("%s", books[index].publisher);
printf("价格:");
scanf("%lf", &books[index].price);
printf("修改成功!\n");
}
}
// 定义函数:查询图书
void searchBook(struct Book books[], int count) {
int id, index = -1;
printf("请输入待查询图书编号:");
scanf("%d", &id);
for (int i = 0; i < count; i++) {
if (books[i].id == id) {
index = i;
break;
}
}
if (index == -1) {
printf("未找到该图书!\n");
} else {
printf("编号\t书名\t作者\t出版社\t价格\n");
printf("%d\t%s\t%s\t%s\t%.2lf\n", books[index].id, books[index].name, books[index].author, books[index].publisher, books[index].price);
}
}
int main() {
struct Book books[100];
int count = 0, choice;
while (1) {
showMenu();
printf("请选择:");
scanf("%d", &choice);
switch (choice) {
case 1:
addBook(books, &count);
break;
case 2:
deleteBook(books, &count);
break;
case 3:
modifyBook(books, count);
break;
case 4:
searchBook(books, count);
break;
case 0:
printf("欢迎下次再来!\n");
exit(0);
default:
printf("输入有误,请重新输入!\n");
break;
}
}
return 0;
}
```
上面的代码实现了一个简单的图书管理系统,包括添加图书、删除图书、修改图书信息和查询图书等功能。您可以根据需要进行修改和完善。
阅读全文
相关推荐
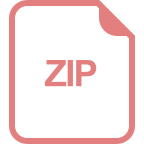
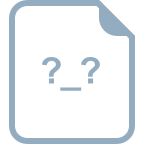
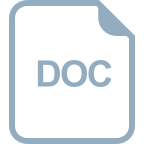
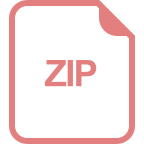
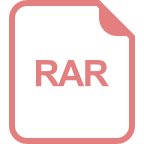
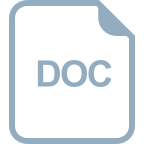





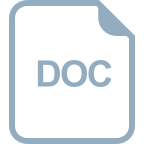
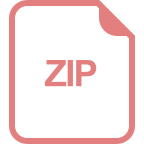
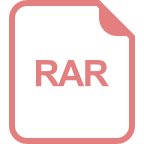
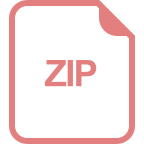