使用系统调用SHMGET(),SHMAT()编制一长度为1K的消息发送和接收的程序。 要求: (1)main函数建立一个KEY 为75 的共享区,并将第一个字节置为0。作为数据空的标志等待其他进程发来的消息。收到数据后显示“ Main recieved help ”。 (2)创建一个进程P1,P1建立一个KEY为75的共享区,当共享取得第一个字节为0时,main空闲,可发送请求:P1填入“HELP”,发送数据后显示“P1 send help”。
时间: 2024-02-25 21:52:29 浏览: 32
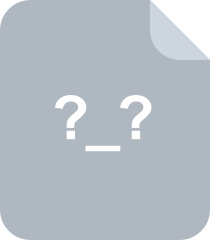
编制一个长度为1K的消息发送和接受的程序。

好的,根据您的要求,我来为您编写一下程序。
首先是发送程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/shm.h>
#define SHM_SIZE 1024
#define SHM_KEY 75
int main() {
int shmid;
char *shmaddr;
char *msg = "HELP";
// 创建共享内存
shmid = shmget(SHM_KEY, SHM_SIZE, IPC_CREAT | 0666);
if (shmid == -1) {
perror("shmget error");
exit(EXIT_FAILURE);
}
// 将共享内存映射到进程地址空间
shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (char *) -1) {
perror("shmat error");
exit(EXIT_FAILURE);
}
// 等待数据空闲
while (*shmaddr != 0);
// 将消息写入共享内存
strcpy(shmaddr, msg);
printf("P1 send help\n");
// 解除映射并删除共享内存
shmdt(shmaddr);
shmctl(shmid, IPC_RMID, NULL);
return 0;
}
```
然后是接收程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/shm.h>
#define SHM_SIZE 1024
#define SHM_KEY 75
int main() {
int shmid;
char *shmaddr;
// 创建共享内存
shmid = shmget(SHM_KEY, SHM_SIZE, IPC_CREAT | 0666);
if (shmid == -1) {
perror("shmget error");
exit(EXIT_FAILURE);
}
// 将共享内存映射到进程地址空间
shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (char *) -1) {
perror("shmat error");
exit(EXIT_FAILURE);
}
// 将第一个字节置为0,作为数据空的标志
*shmaddr = 0;
// 等待其他进程发来的消息
while (1) {
if (*shmaddr != 0) {
printf("Main recieved help\n");
break;
}
}
// 解除映射并删除共享内存
shmdt(shmaddr);
shmctl(shmid, IPC_RMID, NULL);
return 0;
}
```
编译命令:
```
gcc sender.c -o sender
gcc receiver.c -o receiver
```
运行命令:
```
./receiver &
./sender
```
注意,这里使用`&`将接收程序放到后台运行,以便能够同时运行发送程序。
阅读全文
相关推荐
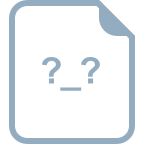




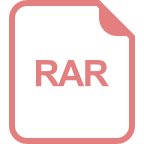
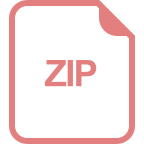
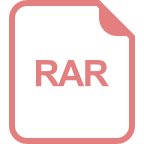
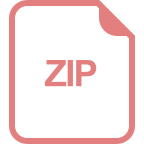
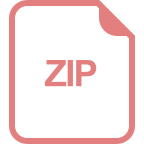
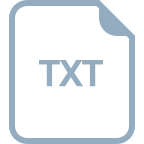
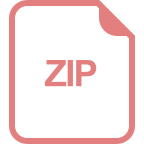
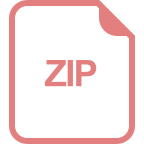