在不使用链表的情况下,用easyx写一个贪吃蛇游戏
时间: 2024-02-01 22:15:28 浏览: 75
好的,下面是一个简单的贪吃蛇游戏的代码,使用了EasyX库:
```c++
#include <graphics.h>
#include <conio.h>
#include <time.h>
#define WIDTH 800 // 窗口宽度
#define HEIGHT 600 // 窗口高度
#define SIZE 20 // 方块大小
struct Node { // 贪吃蛇身体节点结构体
int x, y; // 节点坐标
Node* next; // 下一个节点的指针
};
enum Direction { // 方向枚举类型
UP, DOWN, LEFT, RIGHT
};
int score = 0; // 得分
Node* head = nullptr; // 贪吃蛇头部节点指针
Node* tail = nullptr; // 贪吃蛇尾部节点指针
Direction direction = RIGHT; // 贪吃蛇运动方向
bool isGameOver = false; // 游戏是否结束
void initSnake() { // 初始化贪吃蛇
Node* node = new Node{ WIDTH / 2, HEIGHT / 2, nullptr }; // 创建一个初始节点
head = tail = node; // 初始化头尾指针
setfillcolor(GREEN); // 设置贪吃蛇颜色
solidrectangle(head->x, head->y, head->x + SIZE, head->y + SIZE); // 绘制初始节点
}
void createFood(int& x, int& y) { // 生成食物
srand((unsigned)time(nullptr)); // 随机数种子
x = rand() % (WIDTH / SIZE) * SIZE; // 随机生成横坐标
y = rand() % (HEIGHT / SIZE) * SIZE; // 随机生成纵坐标
setfillcolor(RED); // 设置食物颜色
solidrectangle(x, y, x + SIZE, y + SIZE); // 绘制食物
}
void addNode() { // 添加节点
Node* node = new Node{ tail->x, tail->y, nullptr }; // 创建一个新节点
tail->next = node; // 将新节点连接到尾部
tail = node; // 更新尾部指针
}
void drawScore() { // 绘制得分
setbkmode(TRANSPARENT); // 设置背景透明
settextcolor(BLACK); // 设置文字颜色
settextstyle(24, 0, _T("Consolas")); // 设置字体大小和样式
TCHAR s[10]; // 定义字符串缓冲区
_stprintf_s(s, _T("得分:%d"), score); // 格式化字符串
outtextxy(10, 10, s); // 绘制文字
}
void gameOver() { // 游戏结束
setbkmode(TRANSPARENT); // 设置背景透明
settextcolor(BLACK); // 设置文字颜色
settextstyle(48, 0, _T("Consolas")); // 设置字体大小和样式
outtextxy(WIDTH / 2 - 120, HEIGHT / 2 - 24, _T("游戏结束")); // 绘制文字
isGameOver = true; // 标记游戏结束
}
void updateSnake() { // 更新贪吃蛇
int x = head->x, y = head->y; // 记录头部节点的坐标
switch (direction) { // 根据方向更新头部节点的坐标
case UP:
y -= SIZE;
break;
case DOWN:
y += SIZE;
break;
case LEFT:
x -= SIZE;
break;
case RIGHT:
x += SIZE;
break;
}
if (x < 0 || x >= WIDTH || y < 0 || y >= HEIGHT) { // 判断是否出界
gameOver(); // 游戏结束
return;
}
Node* node = new Node{ x, y, head }; // 创建一个新节点,并将其连接到头部
head = node; // 更新头部指针
setfillcolor(GREEN); // 设置贪吃蛇颜色
solidrectangle(head->x, head->y, head->x + SIZE, head->y + SIZE); // 绘制新节点
if (head->x == foodX && head->y == foodY) { // 判断是否吃到食物
addNode(); // 添加节点
score++; // 增加得分
drawScore(); // 绘制得分
createFood(foodX, foodY); // 生成新的食物
}
else { // 没有吃到食物
setfillcolor(BLACK); // 设置背景颜色
solidrectangle(tail->x, tail->y, tail->x + SIZE, tail->y + SIZE); // 擦除尾部节点
tail = tail->next; // 更新尾部指针
}
for (Node* p = head->next; p != nullptr; p = p->next) { // 判断是否碰到自己
if (head->x == p->x && head->y == p->y) {
gameOver(); // 游戏结束
return;
}
}
}
void handleInput() { // 处理输入
if (_kbhit()) { // 判断是否有按键输入
int key = _getch(); // 获取按键输入
switch (key) {
case 'W':
case 'w':
if (direction != DOWN) // 防止掉头
direction = UP;
break;
case 'S':
case 's':
if (direction != UP)
direction = DOWN;
break;
case 'A':
case 'a':
if (direction != RIGHT)
direction = LEFT;
break;
case 'D':
case 'd':
if (direction != LEFT)
direction = RIGHT;
break;
case 'Q':
case 'q':
gameOver(); // 按下Q键直接结束游戏
break;
}
}
}
int main() {
initgraph(WIDTH, HEIGHT); // 初始化窗口
setbkcolor(WHITE); // 设置背景颜色
initSnake(); // 初始化贪吃蛇
int foodX, foodY; // 食物坐标
createFood(foodX, foodY); // 生成食物
while (!isGameOver) { // 游戏循环
updateSnake(); // 更新贪吃蛇
handleInput(); // 处理输入
Sleep(100); // 等待100毫秒
}
_getch(); // 等待按键结束
closegraph(); // 关闭窗口
return 0;
}
```
上述代码实现了一个简单的贪吃蛇游戏,其中包括贪吃蛇的初始化、食物的生成、节点的添加、得分的绘制、游戏结束的处理等功能。游戏中使用了键盘输入来改变贪吃蛇的运动方向,并且防止了贪吃蛇掉头的情况。
阅读全文
相关推荐
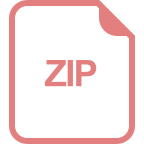
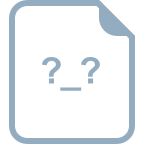
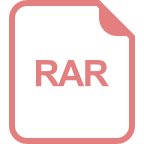
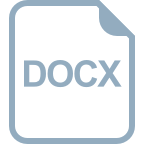
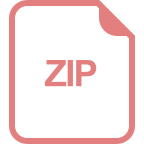
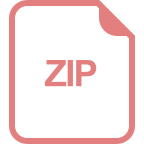
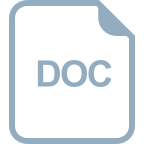
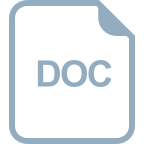
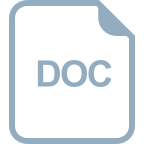
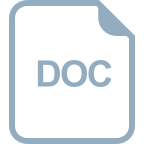
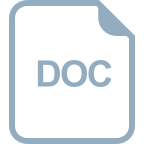

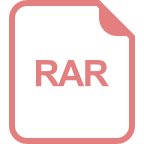
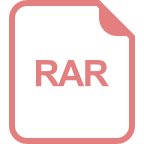
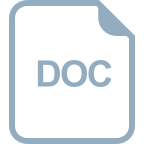
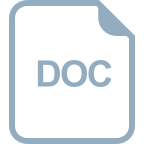
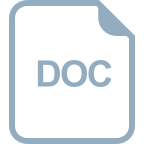
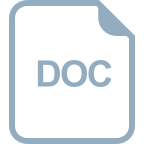