用C++编写旅游客户信息管理系统
时间: 2023-07-11 15:59:08 浏览: 46
好的,旅游客户信息管理系统可以包含以下功能:
1. 添加客户信息:包括客户姓名、性别、年龄、联系方式、身份证号等信息。
2. 删除客户信息:根据客户身份证号或者姓名进行删除。
3. 修改客户信息:可以根据客户身份证号或者姓名进行修改客户的信息。
4. 查询客户信息:可以根据客户身份证号或者姓名进行查询客户的信息。
5. 显示客户信息:将所有客户的信息进行显示。
6. 数据存储:将客户信息进行保存,以便下次使用。
在C++中可以使用结构体来存储客户的信息,代码示例:
```
#include<iostream>
#include<fstream>
#include<cstring>
#include<iomanip>
using namespace std;
struct Customer
{
char name[20]; // 客户姓名
char gender[5]; // 客户性别
int age; // 客户年龄
char phone[20]; // 客户联系方式
char id[20]; // 客户身份证号
};
// 添加客户信息
void addCustomer(Customer customer)
{
// 将客户信息保存到文件中
ofstream outFile("customer.txt", ios::app);
outFile << customer.name << " " << customer.gender << " " << customer.age << " " << customer.phone << " " << customer.id << endl;
outFile.close();
}
// 删除客户信息
void deleteCustomer(char* id)
{
ifstream inFile("customer.txt");
ofstream outFile("temp.txt");
Customer customer;
bool found = false;
while (inFile >> customer.name >> customer.gender >> customer.age >> customer.phone >> customer.id)
{
if (strcmp(id, customer.id) == 0)
{
found = true;
continue;
}
outFile << customer.name << " " << customer.gender << " " << customer.age << " " << customer.phone << " " << customer.id << endl;
}
inFile.close();
outFile.close();
remove("customer.txt");
rename("temp.txt", "customer.txt");
if (!found)
cout << "未找到该客户信息!" << endl;
}
// 修改客户信息
void modifyCustomer(char* id)
{
ifstream inFile("customer.txt");
ofstream outFile("temp.txt");
Customer customer;
bool found = false;
while (inFile >> customer.name >> customer.gender >> customer.age >> customer.phone >> customer.id)
{
if (strcmp(id, customer.id) == 0)
{
found = true;
cout << "请输入客户姓名:";
cin >> customer.name;
cout << "请输入客户性别:";
cin >> customer.gender;
cout << "请输入客户年龄:";
cin >> customer.age;
cout << "请输入客户联系方式:";
cin >> customer.phone;
cout << "请输入客户身份证号:";
cin >> customer.id;
}
outFile << customer.name << " " << customer.gender << " " << customer.age << " " << customer.phone << " " << customer.id << endl;
}
inFile.close();
outFile.close();
remove("customer.txt");
rename("temp.txt", "customer.txt");
if (!found)
cout << "未找到该客户信息!" << endl;
}
// 查询客户信息
void queryCustomer(char* id)
{
ifstream inFile("customer.txt");
Customer customer;
bool found = false;
while (inFile >> customer.name >> customer.gender >> customer.age >> customer.phone >> customer.id)
{
if (strcmp(id, customer.id) == 0)
{
found = true;
cout << "客户姓名:" << customer.name << endl;
cout << "客户性别:" << customer.gender << endl;
cout << "客户年龄:" << customer.age << endl;
cout << "客户联系方式:" << customer.phone << endl;
cout << "客户身份证号:" << customer.id << endl;
break;
}
}
inFile.close();
if (!found)
cout << "未找到该客户信息!" << endl;
}
// 显示所有客户信息
void displayAllCustomers()
{
ifstream inFile("customer.txt");
Customer customer;
cout << left << setw(10) << "姓名" << left << setw(5) << "性别" << left << setw(5) << "年龄" << left << setw(15) << "联系方式" << left << setw(20) << "身份证号" << endl;
while (inFile >> customer.name >> customer.gender >> customer.age >> customer.phone >> customer.id)
{
cout << left << setw(10) << customer.name << left << setw(5) << customer.gender << left << setw(5) << customer.age << left << setw(15) << customer.phone << left << setw(20) << customer.id << endl;
}
inFile.close();
}
int main()
{
int choice;
char id[20];
Customer customer;
while (true)
{
cout << endl << "请选择操作:" << endl;
cout << "1. 添加客户信息" << endl;
cout << "2. 删除客户信息" << endl;
cout << "3. 修改客户信息" << endl;
cout << "4. 查询客户信息" << endl;
cout << "5. 显示客户信息" << endl;
cout << "6. 退出程序" << endl;
cout << "请输入操作编号:";
cin >> choice;
switch (choice)
{
case 1:
cout << "请输入客户姓名:";
cin >> customer.name;
cout << "请输入客户性别:";
cin >> customer.gender;
cout << "请输入客户年龄:";
cin >> customer.age;
cout << "请输入客户联系方式:";
cin >> customer.phone;
cout << "请输入客户身份证号:";
cin >> customer.id;
addCustomer(customer);
break;
case 2:
cout << "请输入客户身份证号或者姓名:";
cin >> id;
deleteCustomer(id);
break;
case 3:
cout << "请输入客户身份证号或者姓名:";
cin >> id;
modifyCustomer(id);
break;
case 4:
cout << "请输入客户身份证号或者姓名:";
cin >> id;
queryCustomer(id);
break;
case 5:
displayAllCustomers();
break;
case 6:
return 0;
default:
cout << "输入错误,请重新输入!" << endl;
break;
}
}
return 0;
}
```
相关推荐














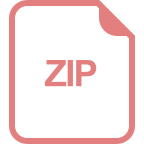
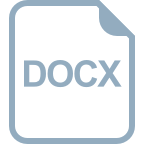