统计数组中出现次数最多的元素
时间: 2023-08-30 19:11:05 浏览: 174
这个问题可以使用哈希表来解决。首先遍历一遍数组,将每个元素出现的次数记录在哈希表中。然后再遍历一遍哈希表,找出出现次数最多的元素即可。
以下是示例代码:
```python
def find_most_frequent_element(arr):
freq_dict = {}
for num in arr:
if num in freq_dict:
freq_dict[num] += 1
else:
freq_dict[num] = 1
max_freq = 0
most_frequent_element = None
for num, freq in freq_dict.items():
if freq > max_freq:
max_freq = freq
most_frequent_element = num
return most_frequent_element
```
该函数接受一个数组作为输入,并返回出现次数最多的元素。
相关问题
统计数组中出现次数最多的元素 用c写
可以使用哈希表来实现统计数组中出现次数最多的元素。具体步骤如下:
1. 定义一个哈希表,用来存储每个元素出现的次数。
2. 遍历数组,将每个元素作为哈希表的键值,出现的次数作为哈希表的值。
3. 遍历哈希表,找到出现次数最多的元素。
下面是具体的 C 语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
struct node {
int key;
int value;
};
struct hashmap {
int size;
struct node **data;
};
struct hashmap *create_map(int size) {
struct hashmap *map = (struct hashmap *)malloc(sizeof(struct hashmap));
map->size = size;
map->data = (struct node **)malloc(sizeof(struct node *) * size);
for (int i = 0; i < size; i++) {
map->data[i] = NULL;
}
return map;
}
int hash_code(struct hashmap *map, int key) {
int code = key % map->size;
if (code < 0) {
code += map->size;
}
return code;
}
void put(struct hashmap *map, int key, int value) {
int code = hash_code(map, key);
while (map->data[code] != NULL && map->data[code]->key != key) {
code = (code + 1) % map->size;
}
if (map->data[code] == NULL) {
struct node *new_node = (struct node *)malloc(sizeof(struct node));
new_node->key = key;
new_node->value = value;
map->data[code] = new_node;
} else {
map->data[code]->value += value;
}
}
int get(struct hashmap *map, int key) {
int code = hash_code(map, key);
while (map->data[code] != NULL && map->data[code]->key != key) {
code = (code + 1) % map->size;
}
if (map->data[code] != NULL) {
return map->data[code]->value;
} else {
return 0;
}
}
void free_map(struct hashmap *map) {
for (int i = 0; i < map->size; i++) {
if (map->data[i] != NULL) {
free(map->data[i]);
}
}
free(map->data);
free(map);
}
int most_frequent(int arr[], int len) {
struct hashmap *map = create_map(MAX_SIZE);
int max_freq = 0;
int max_elem = 0;
for (int i = 0; i < len; i++) {
int freq = get(map, arr[i]);
put(map, arr[i], freq + 1);
if (freq + 1 > max_freq) {
max_freq = freq + 1;
max_elem = arr[i];
}
}
free_map(map);
return max_elem;
}
int main() {
int arr[] = {1, 2, 3, 4, 5, 6, 5, 4, 3, 2, 1, 1};
int len = sizeof(arr) / sizeof(int);
int res = most_frequent(arr, len);
printf("The most frequent element is %d\n", res);
return 0;
}
```
这段代码中,我们首先定义了哈希表的数据结构 `struct hashmap`,其中包含了一个指向节点数组的指针 `data`,以及哈希表的大小 `size`。节点的数据结构 `struct node` 包含了键值 `key` 和值 `value`。
在 `create_map` 函数中,我们创建了一个指定大小的哈希表,并将每个节点初始化为 `NULL`。
在 `hash_code` 函数中,我们使用取模运算将键值映射到哈希表中的索引位置。
在 `put` 函数中,我们首先计算出哈希值,然后遍历节点数组,找到一个空节点或者键值相同的节点,将值加上给定的值。如果没有找到空节点,就创建一个新节点。
在 `get` 函数中,我们首先计算出哈希值,然后遍历节点数组,找到键值相同的节点,返回其值。如果没有找到相同的节点,就返回 0。
在 `most_frequent` 函数中,我们遍历数组,对每个元素进行哈希表操作,更新出现次数最多的元素和其出现次数。最后释放哈希表的内存。
在 `main` 函数中,我们定义了一个数组 `arr`,并调用 `most_frequent` 函数来找到出现次数最多的元素,将其打印出来。
如何使用二分法统计数组中出现次数最多的数字
1. 首先对数组进行排序,可以使用快速排序或归并排序等算法,时间复杂度为O(nlogn)。
2. 定义一个left变量和right变量,分别指向数组的第一个元素和最后一个元素。
3. 在循环中计算中间元素mid,即mid=(left+right)/2。
4. 统计数组中小于等于mid的元素个数count,可以使用二分查找或线性查找。
5. 如果count大于数组长度的一半,说明出现次数最多的数字在左半部分,将right的值更新为mid;否则,出现次数最多的数字在右半部分,将left的值更新为mid+1。
6. 重复执行步骤3-5,直到left等于right,此时出现次数最多的数字就是数组中间的那个数字。
7. 最后再遍历一遍数组,统计该数字出现的次数,如果次数大于数组长度的一半,则返回该数字;否则,返回0表示没有出现次数超过一半的数字。
阅读全文
相关推荐
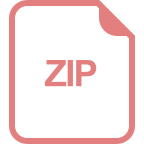
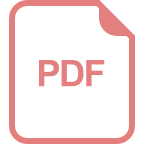
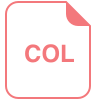












