用string类方法在一个字符串的指定位置插入子串
时间: 2023-10-01 07:10:20 浏览: 195
可以使用string类的insert()方法来在一个字符串的指定位置插入子串。
例如,如果我们有一个字符串s,想要在第3个字符后面插入子串"xyz",可以使用以下代码:
```c++
string s = "abcdefg";
s.insert(3, "xyz");
cout << s << endl;
```
输出结果为:
```
abcxyzdefg
```
其中,insert()方法的第一个参数是插入位置的下标,第二个参数是要插入的子串。
相关问题
定义一个StringUtils类,用String类的方法实现以下函数。 1、修改一个字符串中指定位置的字符。 2、在一个字符串的指定位置插入子串。 3、删除字符串中第一个指定的子串。 4、将字符串反序输出。
1、修改一个字符串中指定位置的字符。
```
public class StringUtils {
public static String replaceChar(String str, int index, char newChar) {
if (str == null || index < 0 || index >= str.length()) {
return str;
}
char[] chars = str.toCharArray();
chars[index] = newChar;
return new String(chars);
}
}
```
2、在一个字符串的指定位置插入子串。
```
public class StringUtils {
public static String insertSubstring(String str, int index, String subStr) {
if (str == null || subStr == null || index < 0 || index > str.length()) {
return str;
}
StringBuilder sb = new StringBuilder(str);
sb.insert(index, subStr);
return sb.toString();
}
}
```
3、删除字符串中第一个指定的子串。
```
public class StringUtils {
public static String removeFirstSubstring(String str, String subStr) {
if (str == null || subStr == null) {
return str;
}
int index = str.indexOf(subStr);
if (index == -1) {
return str;
}
StringBuilder sb = new StringBuilder(str);
sb.delete(index, index + subStr.length());
return sb.toString();
}
}
```
4、将字符串反序输出。
```
public class StringUtils {
public static String reverse(String str) {
if (str == null) {
return str;
}
return new StringBuilder(str).reverse().toString();
}
}
```
java在一个字符串的指定位置插入子串用文字叙述思路以及代码
可以使用StringBuffer或StringBuilder的insert()方法来在字符串的指定位置插入子串。具体思路是:先用substring()方法将原字符串拆分成两个部分,再在两个部分之间插入子串。以下是Java代码示例:
```
String oriStr = "hello world";
String subStr = "beautiful ";
int index = 6; // 在第6个位置插入子串
StringBuilder sb = new StringBuilder(oriStr);
sb.insert(index, subStr);
String newStr = sb.toString();
System.out.println(newStr);
```
这段代码会在原字符串的第6个位置插入 "beautiful " 子串,输出结果为 "hello beautiful world"。需要注意的是,String类型是不可变的,因此我们需要通过StringBuilder或StringBuffer类型进行字符串的修改。
阅读全文
相关推荐








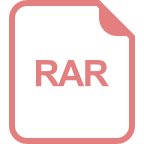






