jsp遍历显示数据库中查询出来的图片
时间: 2024-03-11 20:50:34 浏览: 68
首先,你需要在数据库中存储图片的路径,然后在JSP页面上使用SQL查询语句将路径查询出来,最后使用<img>标签将图片显示出来。
以下是一个简单的JSP页面示例代码,用于遍历显示数据库中查询出来的图片:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<%@ page import="java.sql.*" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>显示图片</title>
</head>
<body>
<h1>显示图片</h1>
<table>
<c:forEach var="item" items="${imageList}">
<tr>
<td>${item.imageName}</td>
<td><img src="${item.imagePath}" alt="${item.imageName}"></td>
</tr>
</c:forEach>
</table>
<%
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/test";
String user = "root";
String password = "root";
conn = DriverManager.getConnection(url, user, password);
String sql = "SELECT * FROM image_table";
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
List<ImageBean> imageList = new ArrayList<ImageBean>();
while (rs.next()) {
ImageBean image = new ImageBean();
image.setImageName(rs.getString("image_name"));
image.setImagePath(rs.getString("image_path"));
imageList.add(image);
}
request.setAttribute("imageList", imageList);
} catch (Exception e) {
e.printStackTrace();
} finally {
if (rs != null) {
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (pstmt != null) {
try {
pstmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (conn != null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
%>
</body>
</html>
```
在上面的示例代码中,我们使用了JSTL的<c:forEach>标签遍历了查询出来的图片列表,然后使用<img>标签将图片显示出来。
同时,我们还在JSP页面中使用了Java代码,通过JDBC连接到数据库中,查询出图片路径并将结果存储在一个List中,最后将List设置为JSP页面的属性,以便在<c:forEach>标签中进行遍历和显示。请注意,为了简化示例代码,我们没有对JDBC连接进行异常处理和资源释放,实际开发中需要注意这些问题。
阅读全文
相关推荐
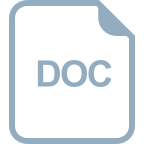
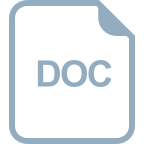
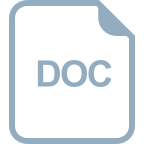


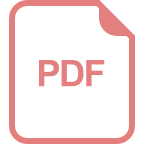
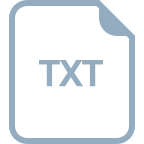
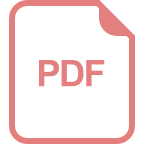
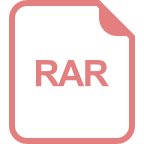
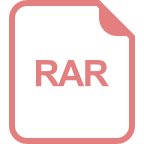
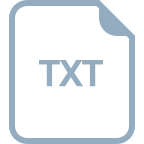
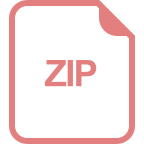
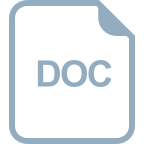
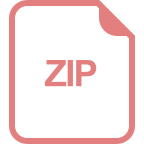
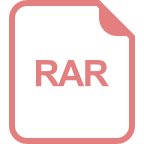
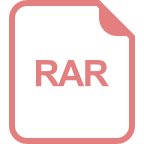
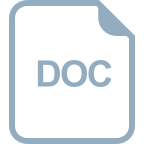
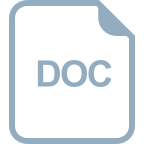
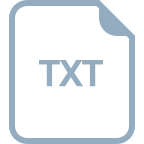