在js中用正则替换字符串
时间: 2023-07-18 21:37:26 浏览: 53
在 JavaScript 中,可以使用 `replace()` 方法和正则表达式来替换字符串。
下面是一个例子:
```javascript
let str = "hello world";
let newStr = str.replace(/hello/, "hi");
console.log(newStr); // 输出: "hi world"
```
在这个例子中,我们使用正则表达式 `/hello/` 匹配字符串中的 "hello",然后用 "hi" 来替换它,最终得到了 "hi world"。注意,这个例子只会替换第一个匹配的字符串。
如果需要替换所有匹配的字符串,可以使用一个全局匹配的正则表达式:
```javascript
let str = "hello hello world";
let newStr = str.replace(/hello/g, "hi");
console.log(newStr); // 输出: "hi hi world"
```
在这个例子中,我们使用正则表达式 `/hello/g` 进行全局匹配,将所有的 "hello" 都替换成了 "hi",最终得到了 "hi hi world"。
相关问题
在js中用正则替换字符串'$$'为换行符
在 JavaScript 中,可以使用 `replace()` 方法和正则表达式来替换字符串中的 `$$` 为换行符。
下面是一个例子:
```javascript
let str = "hello$$world";
let newStr = str.replace(/\$\$/g, "\n");
console.log(newStr); // 输出: "hello\nworld"
```
在这个例子中,我们使用正则表达式 `/\$\$/g` 进行全局匹配,将所有的 `$$` 都替换成了换行符 `\n`,最终得到了 "hello\nworld"。注意,`$` 在正则表达式中具有特殊的含义,需要使用转义符 `\` 来转义。
java中用正则表达式将字符串以空格分开
你可以使用Java中的String类的split()方法和正则表达式来实现将字符串以空格分开。
下面是一个示例代码:
```java
String str = "hello world";
String[] arrOfStr = str.split("\\s+");
for (String a : arrOfStr)
System.out.println(a);
```
输出结果为:
```
hello
world
```
在上面的代码中,我们使用了split()方法并传入一个正则表达式"\\s+"作为参数。这个正则表达式表示一个或多个空格。这将导致split()方法在字符串中找到所有的空格,并将字符串分隔成一个字符串数组。
然后,我们使用for循环遍历数组并输出每个字符串。
相关推荐
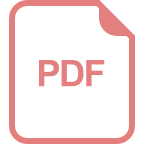
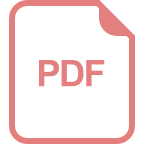
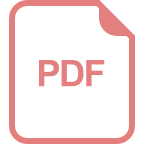












