java导入excel文件校验,将错误的数据标红并下载的代码
时间: 2024-02-15 10:05:22 浏览: 19
以下是一个简单的Java程序,用于从Excel文件中读取数据并校验数据的有效性。如果数据无效,程序将在Excel文件中将其标记为红色,并将其下载到本地计算机。
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.DataFormatter;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
import org.apache.poi.xssf.usermodel.XSSFCellStyle;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelValidation {
public static void main(String[] args) throws IOException {
// 读取Excel文件
FileInputStream fis = new FileInputStream(new File("input.xlsx"));
Workbook workbook = WorkbookFactory.create(fis);
// 获取第一个工作表
Sheet sheet = workbook.getSheetAt(0);
// 创建错误列表
List<Integer> errorList = new ArrayList<Integer>();
// 遍历工作表中的每一行
Iterator<Row> rowIterator = sheet.iterator();
while (rowIterator.hasNext()) {
Row row = rowIterator.next();
// 获取行号
int rowNum = row.getRowNum();
// 获取第一个单元格的值
Cell cell = row.getCell(0);
String value = new DataFormatter().formatCellValue(cell);
// 判断数据有效性
if (value == null || value.trim().isEmpty()) {
errorList.add(rowNum);
}
}
// 如果有错误,则将错误行标记为红色
if (!errorList.isEmpty()) {
// 创建红色单元格样式
XSSFWorkbook xssfWorkbook = new XSSFWorkbook();
XSSFCellStyle redCellStyle = xssfWorkbook.createCellStyle();
redCellStyle.setFillForegroundColor(IndexedColors.RED.getIndex());
redCellStyle.setFillPattern(CellStyle.SOLID_FOREGROUND);
// 遍历错误列表中的每一行,并将其标记为红色
for (Integer rowNum : errorList) {
Row row = sheet.getRow(rowNum);
Cell cell = row.getCell(0);
cell.setCellStyle(redCellStyle);
}
}
// 将工作簿写入新文件
FileOutputStream fos = new FileOutputStream(new File("output.xlsx"));
workbook.write(fos);
fos.close();
// 关闭工作簿和文件输入流
workbook.close();
fis.close();
// 下载文件
// ...
}
}
```
请注意,此代码示例使用Apache POI库来读取和写入Excel文件。如果您尚未安装此库,您需要先安装它才能运行此代码。
相关推荐
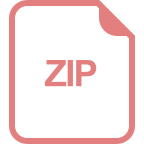
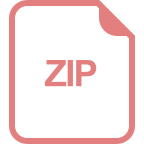












