爬取http://jiuye.scetc.edu.cn/reList招聘信息并写入mysql
时间: 2024-01-30 20:03:31 浏览: 327
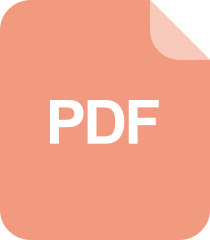
爬取招聘信息
好的,这个任务可以分为两个部分,第一个是爬取网页内容,第二个是将爬取到的内容写入到 MySQL 数据库中。为了实现这个任务,我们需要使用 Python 语言和一些第三方库。
1. 爬取网页内容
我们可以使用 requests 库来获取网页内容,使用 BeautifulSoup 库来解析 HTML。
代码如下:
```python
import requests
from bs4 import BeautifulSoup
url = 'http://jiuye.scetc.edu.cn/reList'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
```
这样就可以获取到网页内容并将其解析为 BeautifulSoup 对象。
2. 写入 MySQL 数据库
我们可以使用 PyMySQL 库来连接 MySQL 数据库,并使用 SQL 语句将数据写入到数据库中。
代码如下:
```python
import pymysql
# 连接 MySQL 数据库
conn = pymysql.connect(host='localhost', user='root', password='password', database='test', charset='utf8mb4')
cursor = conn.cursor()
# 获取数据
items = soup.find_all('div', {'class': 'col-md-12 col-sm-12 col-xs-12 news_item'})
# 将数据写入到数据库中
for item in items:
title = item.find('a').text
href = item.find('a')['href']
date = item.find('span', {'class': 'date'}).text
sql = f"INSERT INTO jobs(title, href, date) VALUES('{title}', '{href}', '{date}')"
cursor.execute(sql)
conn.commit()
conn.close()
```
这样就可以将爬取到的数据写入到 MySQL 数据库中了。
完整代码如下:
```python
import requests
from bs4 import BeautifulSoup
import pymysql
url = 'http://jiuye.scetc.edu.cn/reList'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
# 连接 MySQL 数据库
conn = pymysql.connect(host='localhost', user='root', password='password', database='test', charset='utf8mb4')
cursor = conn.cursor()
# 获取数据
items = soup.find_all('div', {'class': 'col-md-12 col-sm-12 col-xs-12 news_item'})
# 将数据写入到数据库中
for item in items:
title = item.find('a').text
href = item.find('a')['href']
date = item.find('span', {'class': 'date'}).text
sql = f"INSERT INTO jobs(title, href, date) VALUES('{title}', '{href}', '{date}')"
cursor.execute(sql)
conn.commit()
conn.close()
```
需要注意的是,我们需要将代码中的数据库连接信息修改为自己的数据库信息。
阅读全文
相关推荐
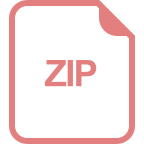
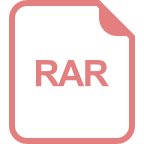
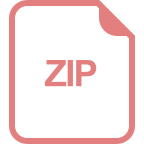
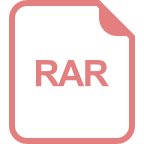
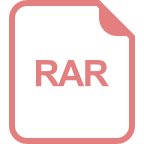
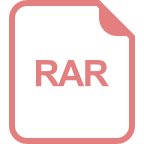
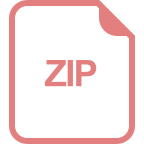
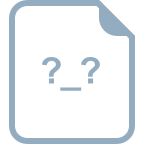
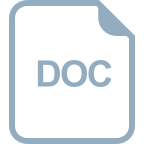
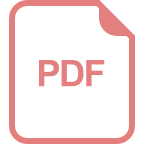
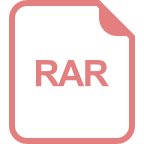
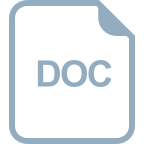
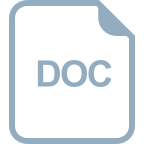
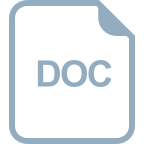


