编写 Scala 工程代码,将 ods 库中表 table1、table2、table3、table4、 table5、table6、table7、table8、table9、table10、table11 抽取到 Hive 的 dwd 库中对应表中。表中有涉及到 timestamp 类型的,均要求按照 yyyy-MM-dd HH:mm:ss,不记录毫秒数,若原数据中只有年月日,则在时分秒的位置添加 00:00:00,添加之后使其符合 yyyy-MM-dd HH:mm:ss。
时间: 2023-06-16 09:03:34 浏览: 230
以下是一个可能的 Scala 工程代码示例,用于将 ods 库中的表抽取到 Hive 的 dwd 库中,同时格式化 timestamp 类型的数据:
```scala
import java.text.SimpleDateFormat
import java.util.{Calendar, TimeZone}
import org.apache.spark.sql.SparkSession
import org.apache.spark.sql.functions._
object OdsToDwd {
def main(args: Array[String]): Unit = {
val spark = SparkSession.builder()
.appName("OdsToDwd")
.enableHiveSupport()
.getOrCreate()
// 从 ods 库中读取表数据
val table1 = spark.table("ods.table1")
val table2 = spark.table("ods.table2")
val table3 = spark.table("ods.table3")
val table4 = spark.table("ods.table4")
val table5 = spark.table("ods.table5")
val table6 = spark.table("ods.table6")
val table7 = spark.table("ods.table7")
val table8 = spark.table("ods.table8")
val table9 = spark.table("ods.table9")
val table10 = spark.table("ods.table10")
val table11 = spark.table("ods.table11")
// 格式化 timestamp 类型数据
val dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss")
dateFormat.setTimeZone(TimeZone.getTimeZone("UTC"))
val formatTimestamp = udf((timestamp: String) => {
if (timestamp == null) {
null
} else {
val cal = Calendar.getInstance(TimeZone.getTimeZone("UTC"))
cal.setTimeInMillis(timestamp.toLong)
cal.set(Calendar.MILLISECOND, 0)
dateFormat.format(cal.getTime)
}
})
// 将数据写入 dwd 库中对应表
table1.selectExpr("*", "CAST(unix_timestamp(timestamp) AS STRING) AS timestamp_str")
.withColumn("formatted_timestamp", formatTimestamp(col("timestamp_str")))
.drop("timestamp_str")
.write.insertInto("dwd.table1")
table2.selectExpr("*", "CAST(unix_timestamp(timestamp) AS STRING) AS timestamp_str")
.withColumn("formatted_timestamp", formatTimestamp(col("timestamp_str")))
.drop("timestamp_str")
.write.insertInto("dwd.table2")
table3.selectExpr("*", "CAST(unix_timestamp(timestamp) AS STRING) AS timestamp_str")
.withColumn("formatted_timestamp", formatTimestamp(col("timestamp_str")))
.drop("timestamp_str")
.write.insertInto("dwd.table3")
table4.selectExpr("*", "CAST(unix_timestamp(timestamp) AS STRING) AS timestamp_str")
.withColumn("formatted_timestamp", formatTimestamp(col("timestamp_str")))
.drop("timestamp_str")
.write.insertInto("dwd.table4")
table5.selectExpr("*", "CAST(unix_timestamp(timestamp) AS STRING) AS timestamp_str")
.withColumn("formatted_timestamp", formatTimestamp(col("timestamp_str")))
.drop("timestamp_str")
.write.insertInto("dwd.table5")
table6.selectExpr("*", "CAST(unix_timestamp(timestamp) AS STRING) AS timestamp_str")
.withColumn("formatted_timestamp", formatTimestamp(col("timestamp_str")))
.drop("timestamp_str")
.write.insertInto("dwd.table6")
table7.selectExpr("*", "CAST(unix_timestamp(timestamp) AS STRING) AS timestamp_str")
.withColumn("formatted_timestamp", formatTimestamp(col("timestamp_str")))
.drop("timestamp_str")
.write.insertInto("dwd.table7")
table8.selectExpr("*", "CAST(unix_timestamp(timestamp) AS STRING) AS timestamp_str")
.withColumn("formatted_timestamp", formatTimestamp(col("timestamp_str")))
.drop("timestamp_str")
.write.insertInto("dwd.table8")
table9.selectExpr("*", "CAST(unix_timestamp(timestamp) AS STRING) AS timestamp_str")
.withColumn("formatted_timestamp", formatTimestamp(col("timestamp_str")))
.drop("timestamp_str")
.write.insertInto("dwd.table9")
table10.selectExpr("*", "CAST(unix_timestamp(timestamp) AS STRING) AS timestamp_str")
.withColumn("formatted_timestamp", formatTimestamp(col("timestamp_str")))
.drop("timestamp_str")
.write.insertInto("dwd.table10")
table11.selectExpr("*", "CAST(unix_timestamp(timestamp) AS STRING) AS timestamp_str")
.withColumn("formatted_timestamp", formatTimestamp(col("timestamp_str")))
.drop("timestamp_str")
.write.insertInto("dwd.table11")
}
}
```
需要注意的是,这只是一个示例,实际代码可能需要根据具体情况进行修改和调整。
阅读全文
相关推荐
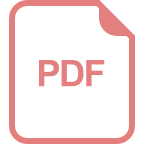
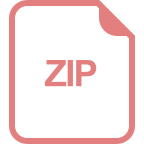
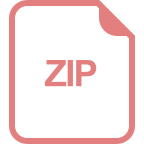














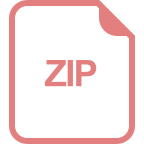