用c++写一个mysql的超市管理系统
时间: 2023-12-04 12:05:45 浏览: 46
这里提供一个简单的超市管理系统的C++代码,使用MySQL数据库进行数据存储。
注:本代码基于 Windows 操作系统,需要安装 MySQL Connector/C++ 和 MySQL Server,并且需要在 MySQL Server 中创建名为 "supermarket" 的数据库,以及名为 "goods" 的数据表。
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <mysql_connection.h>
#include <mysql_driver.h>
#include <cppconn/statement.h>
#include <cppconn/prepared_statement.h>
#include <cppconn/resultset.h>
using namespace std;
using namespace sql;
// 数据库连接信息
const string SERVER = "localhost";
const string USER = "root";
const string PASSWORD = "123456";
const string DATABASE = "supermarket";
// 商品信息结构体
struct GoodsInfo {
int id;
string name;
double price;
int count;
};
// 添加商品
void AddGoods(Connection* conn) {
string name;
double price;
int count;
cout << "请输入商品名称:";
cin >> name;
cout << "请输入商品单价:";
cin >> price;
cout << "请输入商品数量:";
cin >> count;
PreparedStatement* pstmt = conn->prepareStatement("INSERT INTO goods(name, price, count) VALUES (?, ?, ?)");
pstmt->setString(1, name);
pstmt->setDouble(2, price);
pstmt->setInt(3, count);
pstmt->executeUpdate();
delete pstmt;
cout << "添加成功!" << endl << endl;
}
// 修改商品
void ModifyGoods(Connection* conn) {
int id;
double price;
int count;
cout << "请输入要修改的商品编号:";
cin >> id;
PreparedStatement* pstmt = conn->prepareStatement("SELECT * FROM goods WHERE id = ?");
pstmt->setInt(1, id);
ResultSet* rs = pstmt->executeQuery();
if (rs->next()) {
cout << "当前商品信息如下:" << endl;
cout << "编号:" << rs->getInt("id") << endl;
cout << "名称:" << rs->getString("name") << endl;
cout << "单价:" << rs->getDouble("price") << endl;
cout << "数量:" << rs->getInt("count") << endl;
cout << "请输入新单价:";
cin >> price;
cout << "请输入新数量:";
cin >> count;
delete rs;
delete pstmt;
pstmt = conn->prepareStatement("UPDATE goods SET price = ?, count = ? WHERE id = ?");
pstmt->setDouble(1, price);
pstmt->setInt(2, count);
pstmt->setInt(3, id);
pstmt->executeUpdate();
delete pstmt;
cout << "修改成功!" << endl << endl;
}
else {
cout << "商品不存在!" << endl << endl;
}
}
// 删除商品
void DeleteGoods(Connection* conn) {
int id;
cout << "请输入要删除的商品编号:";
cin >> id;
PreparedStatement* pstmt = conn->prepareStatement("SELECT * FROM goods WHERE id = ?");
pstmt->setInt(1, id);
ResultSet* rs = pstmt->executeQuery();
if (rs->next()) {
delete rs;
delete pstmt;
pstmt = conn->prepareStatement("DELETE FROM goods WHERE id = ?");
pstmt->setInt(1, id);
pstmt->executeUpdate();
delete pstmt;
cout << "删除成功!" << endl << endl;
}
else {
cout << "商品不存在!" << endl << endl;
}
}
// 查询商品
void QueryGoods(Connection* conn) {
PreparedStatement* pstmt = conn->prepareStatement("SELECT * FROM goods");
ResultSet* rs = pstmt->executeQuery();
vector<GoodsInfo> goodsList;
while (rs->next()) {
GoodsInfo goods;
goods.id = rs->getInt("id");
goods.name = rs->getString("name");
goods.price = rs->getDouble("price");
goods.count = rs->getInt("count");
goodsList.push_back(goods);
}
delete rs;
delete pstmt;
if (goodsList.size() > 0) {
cout << "编号\t名称\t单价\t数量" << endl;
for (int i = 0; i < goodsList.size(); i++) {
GoodsInfo goods = goodsList[i];
cout << goods.id << "\t" << goods.name << "\t" << goods.price << "\t" << goods.count << endl;
}
cout << endl;
}
else {
cout << "暂无商品信息!" << endl << endl;
}
}
int main() {
try {
// 连接数据库
Driver* driver = get_driver_instance();
Connection* conn = driver->connect("tcp://" + SERVER, USER, PASSWORD);
conn->setSchema(DATABASE);
// 菜单循环
while (true) {
cout << "超市管理系统" << endl;
cout << "1. 添加商品" << endl;
cout << "2. 修改商品" << endl;
cout << "3. 删除商品" << endl;
cout << "4. 查询商品" << endl;
cout << "0. 退出系统" << endl;
cout << "请选择操作:";
int choice;
cin >> choice;
switch (choice) {
case 1:
AddGoods(conn);
break;
case 2:
ModifyGoods(conn);
break;
case 3:
DeleteGoods(conn);
break;
case 4:
QueryGoods(conn);
break;
case 0:
cout << "谢谢使用!" << endl;
return 0;
default:
cout << "输入错误,请重新选择!" << endl << endl;
break;
}
}
conn->close();
delete conn;
}
catch (SQLException& e) {
cout << "数据库连接异常:" << e.what() << endl;
}
return 0;
}
```
希望这个代码能够帮助到你!
相关推荐
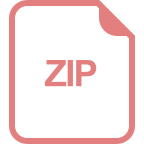
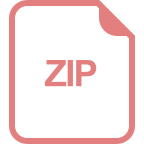














