如何用c++写一个超市库存管理函数
时间: 2023-06-26 22:05:55 浏览: 96
下面是一个简单的超市库存管理函数的例子,它使用了C++的类来实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Product {
public:
string name;
int quantity;
double price;
// 构造函数
Product(string n, int q, double p) {
name = n;
quantity = q;
price = p;
}
// 输出商品信息
void printInfo() {
cout << "Name: " << name << endl;
cout << "Quantity: " << quantity << endl;
cout << "Price: " << price << endl;
}
};
class Inventory {
private:
Product* products[100]; // 最多100种商品
int numProducts; // 当前商品数量
public:
// 构造函数
Inventory() {
numProducts = 0;
}
// 添加商品
void addProduct(Product* p) {
products[numProducts] = p;
numProducts++;
}
// 根据名称查找商品
Product* findProduct(string name) {
for (int i = 0; i < numProducts; i++) {
if (products[i]->name == name) {
return products[i];
}
}
return nullptr;
}
// 更新商品数量
void updateQuantity(string name, int quantity) {
Product* p = findProduct(name);
if (p != nullptr) {
p->quantity = quantity;
}
}
// 输出所有商品信息
void printInventory() {
for (int i = 0; i < numProducts; i++) {
products[i]->printInfo();
cout << endl;
}
}
};
int main() {
// 初始化超市库存
Inventory inventory;
Product* p1 = new Product("Apple", 10, 2.5);
Product* p2 = new Product("Orange", 20, 1.5);
inventory.addProduct(p1);
inventory.addProduct(p2);
// 输出所有商品信息
inventory.printInventory();
// 更新商品数量
inventory.updateQuantity("Apple", 15);
// 输出更新后的商品信息
inventory.printInventory();
return 0;
}
```
这个例子中,我们使用了两个类:`Product` 和 `Inventory`。`Product` 类表示一个商品,包含名称、数量和价格等属性;`Inventory` 类表示整个超市的库存,包含一个商品数组和一些针对商品的操作,比如添加商品、查找商品和更新商品数量等。
在 `main` 函数中,我们首先创建了两个商品 `p1` 和 `p2`,然后将它们添加到 `inventory` 实例中。接着,我们输出了所有商品信息,然后更新了苹果的数量,最后再次输出所有商品信息。
当然,这只是一个简单的例子,实际的库存管理系统可能会更加复杂。例如,我们可能需要支持从文件中读取商品信息,或者支持多种货币的价格计算。但是,这个例子可以帮助你了解如何用 C++ 实现一个基本的库存管理函数。
阅读全文
相关推荐
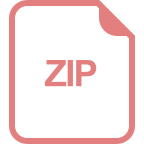
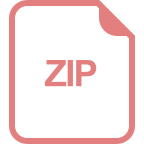





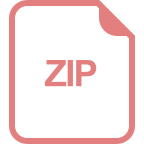
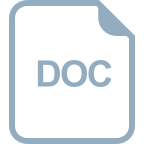
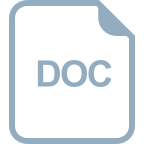







