用C++写一个超市管理系统
时间: 2023-12-27 22:45:45 浏览: 28
以下是一个简单的超市管理系统的C++代码示例:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
// 商品结构体
struct Product {
string name;
double price;
int quantity;
};
// 超市管理类
class Supermarket {
private:
vector<Product> products; // 商品列表
public:
// 从文件中读取商品信息
void loadProducts() {
ifstream file("products.txt");
if (file.is_open()) {
string name;
double price;
int quantity;
while (file >> name >> price >> quantity) {
Product product = { name, price, quantity };
products.push_back(product);
}
file.close();
}
}
// 展示商品列表
void displayProducts() {
cout << "商品列表:" << endl;
for (int i = 0; i < products.size(); i++) {
cout << i + 1 << ". " << products[i].name << " - ¥" << products[i].price << " - 库存:" << products[i].quantity << endl;
}
}
// 添加商品
void addProduct() {
cout << "请输入商品名称:";
string name;
cin >> name;
cout << "请输入商品价格:";
double price;
cin >> price;
cout << "请输入商品库存:";
int quantity;
cin >> quantity;
Product product = { name, price, quantity };
products.push_back(product);
cout << "添加成功!" << endl;
}
// 删除商品
void deleteProduct() {
cout << "请输入要删除的商品编号:";
int index;
cin >> index;
if (index > 0 && index <= products.size()) {
products.erase(products.begin() + index - 1);
cout << "删除成功!" << endl;
}
else {
cout << "无效的商品编号!" << endl;
}
}
// 修改商品信息
void editProduct() {
cout << "请输入要修改的商品编号:";
int index;
cin >> index;
if (index > 0 && index <= products.size()) {
Product product = products[index - 1];
cout << "请输入新的商品名称(原名称为 " << product.name << "):";
cin >> product.name;
cout << "请输入新的商品价格(原价格为 " << product.price << "):";
cin >> product.price;
cout << "请输入新的商品库存(原库存为 " << product.quantity << "):";
cin >> product.quantity;
products[index - 1] = product;
cout << "修改成功!" << endl;
}
else {
cout << "无效的商品编号!" << endl;
}
}
// 商品销售
void sellProduct() {
cout << "请输入要售出的商品编号:";
int index;
cin >> index;
if (index > 0 && index <= products.size()) {
Product product = products[index - 1];
int quantity;
cout << "请输入要售出的数量(当前库存为 " << product.quantity << "):";
cin >> quantity;
if (quantity > 0 && quantity <= product.quantity) {
product.quantity -= quantity;
products[index - 1] = product;
cout << "售出成功!" << endl;
}
else {
cout << "无效的数量!" << endl;
}
}
else {
cout << "无效的商品编号!" << endl;
}
}
// 保存商品信息到文件
void saveProducts() {
ofstream file("products.txt");
if (file.is_open()) {
for (int i = 0; i < products.size(); i++) {
file << products[i].name << " " << products[i].price << " " << products[i].quantity << endl;
}
file.close();
}
}
};
int main() {
Supermarket supermarket;
supermarket.loadProducts(); // 从文件中读取商品信息
while (true) {
cout << "请选择操作:" << endl;
cout << "1. 展示商品列表" << endl;
cout << "2. 添加商品" << endl;
cout << "3. 删除商品" << endl;
cout << "4. 修改商品信息" << endl;
cout << "5. 商品销售" << endl;
cout << "6. 退出程序" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
supermarket.displayProducts();
break;
case 2:
supermarket.addProduct();
break;
case 3:
supermarket.deleteProduct();
break;
case 4:
supermarket.editProduct();
break;
case 5:
supermarket.sellProduct();
break;
case 6:
supermarket.saveProducts(); // 将商品信息保存到文件
return 0;
default:
cout << "无效的选择!" << endl;
break;
}
}
}
```
在这个示例中,超市管理系统使用了一个`Supermarket`类来管理商品。它包含了一系列方法:`loadProducts`用于从文件中读取商品信息,`displayProducts`用于展示商品列表,`addProduct`用于添加商品,`deleteProduct`用于删除商品,`editProduct`用于修改商品信息,`sellProduct`用于销售商品,以及`saveProducts`用于将商品信息保存到文件。
在`main`函数中,我们首先调用`loadProducts`方法从文件中读取商品信息,然后进入一个循环,不断接受用户的输入并调用相应的方法来执行操作。当用户选择退出程序时,我们调用`saveProducts`方法将商品信息保存到文件中。
需要注意的是,这个示例仅仅展示了一个简单的超市管理系统,实际的超市管理系统可能需要更复杂的功能和更多的数据管理。
相关推荐
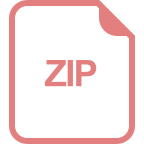














