输入字符串,仅包含小写字母,输出字符串中出现次数最多的字母及其出现次数。只输出第一个次数最多的字母及次数
时间: 2023-10-30 11:04:43 浏览: 29
好的,这是一个简单的字符串统计问题,可以使用哈希表来解决。以下是示例Python代码:
```python
def find_most_common_char(s):
# 初始化哈希表
char_count = {}
# 遍历字符串,统计每个字符出现次数
for c in s:
if c in char_count:
char_count[c] += 1
else:
char_count[c] = 1
# 找出出现次数最多的字符及其出现次数
max_count = 0
max_char = ''
for c, count in char_count.items():
if count > max_count:
max_count = count
max_char = c
return max_char, max_count
```
你可以通过调用 `find_most_common_char` 函数来解决这个问题。例如,对于输入字符串 "abcbcbb",该函数将返回 ('b', 4)。
相关问题
python:输入字符串,仅包含小写字母,输出字符串中出现次数最多的字母及其出现次数。只输出第一个次数最多的字母及次数。
可以使用Python中的collections模块中的Counter类来实现:
```python
from collections import Counter
s = input()
c = Counter(s)
most_common = c.most_common(1)[0]
print(most_common[0], most_common[1])
```
输入一个字符串,然后使用Counter类统计每个字母出现的次数,再使用most_common方法找到出现次数最多的字母及其出现次数,最后输出即可。
python实现输入字符串,仅包含小写字母,输出字符串中出现次数最多的字母及其出现次数。只输出第一个次数最多的字母及次数。
可以使用Python内置的collections模块中的Counter类来实现。具体的代码如下:
```python
from collections import Counter
s = input("请输入字符串:")
# 统计每个字母出现的次数
count = Counter(s)
# 找到出现次数最多的字母及其出现次数
most_common, most_common_count = count.most_common(1)[0]
print("出现次数最多的字母是 {},出现次数为 {}".format(most_common, most_common_count))
```
这里使用`Counter(s)`统计每个字母出现的次数,然后调用`most_common(1)`方法找到出现次数最多的字母及其出现次数,最后输出结果。
阅读全文
相关推荐
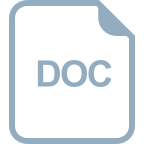
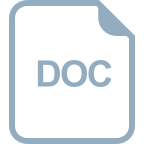
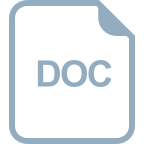






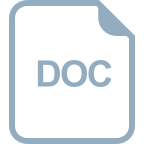
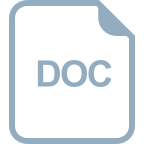
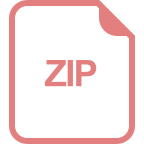
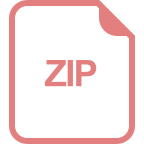
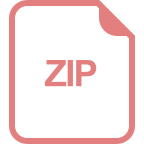