用java实现基于cs模式的sm4算法加解密软件实现
时间: 2023-12-10 13:03:47 浏览: 29
好的,以下是Java实现的基于cs模式的sm4算法加解密软件实现:
服务端代码:
```java
import cn.com.sgcc.crypto.SM4;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
import java.nio.charset.StandardCharsets;
public class SM4Server {
private static final int PORT = 8888;
private static final String KEY = "1234567890abcdef";
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(PORT);
System.out.println("Server started, waiting for client connection...");
while (true) {
Socket socket = serverSocket.accept();
System.out.println("Client connected: " + socket.getInetAddress().getHostAddress());
new Thread(() -> {
try {
DataInputStream dis = new DataInputStream(socket.getInputStream());
DataOutputStream dos = new DataOutputStream(socket.getOutputStream());
while (true) {
int type = dis.readInt();
if (type == 0) { // 加密
int length = dis.readInt();
byte[] data = new byte[length];
dis.readFully(data);
byte[] encryptedData = SM4.encrypt_ECB(data, KEY.getBytes(StandardCharsets.UTF_8));
dos.writeInt(encryptedData.length);
dos.write(encryptedData);
dos.flush();
} else if (type == 1) { // 解密
int length = dis.readInt();
byte[] data = new byte[length];
dis.readFully(data);
byte[] decryptedData = SM4.decrypt_ECB(data, KEY.getBytes(StandardCharsets.UTF_8));
dos.writeInt(decryptedData.length);
dos.write(decryptedData);
dos.flush();
} else {
break;
}
}
dis.close();
dos.close();
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}).start();
}
}
}
```
客户端代码:
```java
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.IOException;
import java.net.Socket;
import java.nio.charset.StandardCharsets;
public class SM4Client {
private static final String HOST = "127.0.0.1";
private static final int PORT = 8888;
public static void main(String[] args) throws IOException {
Socket socket = new Socket(HOST, PORT);
System.out.println("Connected to server: " + socket.getInetAddress().getHostAddress());
DataInputStream dis = new DataInputStream(socket.getInputStream());
DataOutputStream dos = new DataOutputStream(socket.getOutputStream());
String input = "这是一段测试字符串。";
byte[] inputData = input.getBytes(StandardCharsets.UTF_8);
// 加密
dos.writeInt(0); // 指定为加密操作
dos.writeInt(inputData.length);
dos.write(inputData);
dos.flush();
int encryptedLength = dis.readInt();
byte[] encryptedData = new byte[encryptedLength];
dis.readFully(encryptedData);
String encryptedText = new String(encryptedData, StandardCharsets.UTF_8);
System.out.println("Encrypted text: " + encryptedText);
// 解密
dos.writeInt(1); // 指定为解密操作
dos.writeInt(encryptedLength);
dos.write(encryptedData);
dos.flush();
int decryptedLength = dis.readInt();
byte[] decryptedData = new byte[decryptedLength];
dis.readFully(decryptedData);
String decryptedText = new String(decryptedData, StandardCharsets.UTF_8);
System.out.println("Decrypted text: " + decryptedText);
dis.close();
dos.close();
socket.close();
}
}
```
需要注意的是,在这里我们使用了SM4算法的ECB模式进行加解密,因此需要保证每次加密的明文长度和密钥长度是一致的。在实际应用中,可以考虑使用SM4算法的CBC或CTR模式,以提高加密的安全性。同时,在实际应用中还需要考虑对数据进行完整性校验,防止数据被篡改。
相关推荐
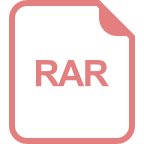
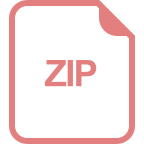














