3)On the basis of question 2, rewrite the C program to shift the sequence to the right by n bits. Sequence input, sequence output and sequence right shift are realized by three sub functions respectively. (The number of series and the number of bits shifted to the right are entered by the user from the keyboard) For example: Enter the length of the sequence:6 Input original sequence:1 2 3 4 5 6 Enter the number of digits shifted to the right:3 After the cycle shifts 3 bits to the right, it becomes: 4 5 6 1 2 3
时间: 2024-01-05 21:03:06 浏览: 27
Sure, I can help you with that! Here's the modified C program:
```c
#include <stdio.h>
// Function to read the sequence from the user
void readSequence(int sequence[], int length) {
printf("Input original sequence:");
for (int i = 0; i < length; i++) {
scanf("%d", &sequence[i]);
}
}
// Function to print the shifted sequence
void printSequence(int sequence[], int length) {
printf("After the cycle shifts %d bits to the right, it becomes:", length);
for (int i = 0; i < length; i++) {
printf(" %d", sequence[i]);
}
printf("\n");
}
// Function to shift the sequence to the right by n bits
void shiftSequence(int sequence[], int length, int n) {
int temp;
for (int i = 0; i < n; i++) {
// Store the last element of the sequence in a temporary variable
temp = sequence[length - 1];
// Shift all elements to the right by one position
for (int j = length - 1; j > 0; j--) {
sequence[j] = sequence[j - 1];
}
// Place the last element at the beginning of the sequence
sequence[0] = temp;
}
}
int main() {
int length, n;
printf("Enter the length of the sequence:");
scanf("%d", &length);
int sequence[length];
readSequence(sequence, length);
printf("Enter the number of digits shifted to the right:");
scanf("%d", &n);
shiftSequence(sequence, length, n);
printSequence(sequence, length);
return 0;
}
```
I hope this helps! Let me know if you have any questions.
相关推荐
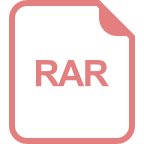
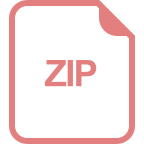
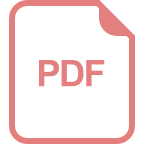














