实现用pytoch框架实现基于深度神经网络的手写数字识别模型
时间: 2023-02-06 15:20:35 浏览: 66
在 PyTorch 中实现基于深度神经网络的手写数字识别模型,需要执行以下步骤:
1. 准备训练数据和测试数据,这通常需要使用某种数据集,例如MNIST或CIFAR-10。这些数据集通常包含许多手写数字图像和相应的标签,其中每个标签都指示图像中手写的数字。
2. 定义模型架构。这通常包括定义输入层、隐藏层和输出层的数量和结构。为了实现深度神经网络,通常需要定义至少一个隐藏层。
3. 选择损失函数和优化器。对于手写数字识别模型,通常使用交叉熵损失函数。优化器则用于更新模型的参数,以便最小化损失函数。常用的优化器包括随机梯度下降(SGD)和Adam。
4. 定义训练循环。在训练循环中,需要通过将批量的训练数据输入模型并计算损失来训练模型。然后,使用优化器更新模型的参数,以便最小化损失。训练循环应该在多个 epoch(即对数据的完整遍历)之间进行
相关问题
pytorch基于卷积神经网络的手写数字识别GUI界面搭建
好的,这是一个很有趣的问题,我来给您解答。
首先,我们需要训练一个卷积神经网络模型来实现手写数字识别。您可以使用PyTorch深度学习框架来实现这个模型。您可以使用MNIST数据集进行训练。在训练完成后,您需要将模型保存下来以备后续使用。
接下来,我们需要搭建一个GUI界面。您可以使用Python的Tkinter库来实现一个基本的GUI界面。在界面中,您可以添加一个画布,让用户在上面手写数字。然后,您需要使用Python的Pillow库将画布中的图像转换成28x28的灰度图像,并将其传递给卷积神经网络模型进行预测。最后,您可以将预测结果显示在GUI界面上。
下面是一个示例代码,供您参考:
```python
import tkinter as tk
from PIL import Image, ImageDraw
import torch
from torchvision import transforms
# 加载训练好的模型
model = torch.load('model.pth')
# 定义图像转换
transform = transforms.Compose([
transforms.Grayscale(),
transforms.Resize((28, 28)),
transforms.ToTensor(),
transforms.Normalize((0.1307,), (0.3081,))
])
# 定义GUI界面
class App:
def __init__(self, master):
self.master = master
self.canvas = tk.Canvas(master, width=200, height=200, bg='white')
self.canvas.pack()
self.canvas.bind('<B1-Motion>', self.draw)
self.button = tk.Button(master, text='识别', command=self.predict)
self.button.pack()
self.label = tk.Label(master, text='')
self.label.pack()
# 在画布上绘制
def draw(self, event):
x, y = event.x, event.y
r = 8
self.canvas.create_oval(x-r, y-r, x+r, y+r, fill='black')
# 图像预处理和预测
def predict(self):
# 获取画布图像
x0 = self.master.winfo_rootx() + self.canvas.winfo_x()
y0 = self.master.winfo_rooty() + self.canvas.winfo_y()
x1 = x0 + self.canvas.winfo_width()
y1 = y0 + self.canvas.winfo_height()
image = ImageGrab.grab((x0, y0, x1, y1))
# 图像预处理
image = transform(image)
# 图像预测
with torch.no_grad():
output = model(image.unsqueeze(0))
pred = output.argmax(dim=1, keepdim=True)
# 显示预测结果
self.label.config(text='预测结果: {}'.format(pred.item()))
# 运行GUI界面
root = tk.Tk()
app = App(root)
root.mainloop()
```
请注意,这只是一个基本的示例代码,您可以根据自己的需求进行修改和扩展。
使用pytorch搭建卷积神经网络识别手写数字
### 回答1:
使用PyTorch搭建卷积神经网络可以很容易地实现手写数字识别。首先需要导入PyTorch库,并加载手写数字数据集。然后,可以定义卷积神经网络的结构,包括卷积层、池化层、全连接层等。接着,可以定义损失函数和优化器,并进行模型训练。最后,可以使用测试集对模型进行评估。整个过程需要注意超参数的选择和调整,以达到最佳的识别效果。
### 回答2:
Pytorch是一个非常流行的深度学习框架,它的设计目的是为了能够快速地搭建神经网络模型,并进行训练和测试。本文将介绍如何使用Pytorch搭建卷积神经网络来对手写数字进行识别。
首先,我们需要准备手写数字数据集,其中包含许多手写数字图片和其对应的标签。这里我们可以使用MNIST数据集,它是一个非常著名的手写数字识别数据集,包含60000张训练图片和10000张测试图片。Pytorch已经内置了该数据集。
接着,我们需要构建卷积神经网络模型。对于手写数字识别任务,我们可以采用经典的LeNet-5模型,它是一个两层卷积层和三层全连接层的模型。在Pytorch中,我们可以使用nn.Module类来定义模型。
模型定义如下:
```
import torch.nn as nn
class LeNet(nn.Module):
def __init__(self):
super(LeNet, self).__init__()
self.conv1 = nn.Conv2d(1, 6, 5)
self.pool1 = nn.MaxPool2d(2)
self.conv2 = nn.Conv2d(6, 16, 5)
self.pool2 = nn.MaxPool2d(2)
self.fc1 = nn.Linear(16 * 4 * 4, 120)
self.fc2 = nn.Linear(120, 84)
self.fc3 = nn.Linear(84, 10)
def forward(self, x):
x = self.conv1(x)
x = nn.functional.relu(x)
x = self.pool1(x)
x = self.conv2(x)
x = nn.functional.relu(x)
x = self.pool2(x)
x = x.view(-1, 16 * 4 * 4)
x = self.fc1(x)
x = nn.functional.relu(x)
x = self.fc2(x)
x = nn.functional.relu(x)
x = self.fc3(x)
return x
```
上述代码定义了一个名为LeNet的模型,该模型由两个卷积层、两个最大池化层和三个全连接层组成,并且采用ReLU作为激活函数。
接下来,我们需要定义损失函数和优化器。在这里,我们将采用交叉熵作为损失函数,优化器使用随机梯度下降(SGD)。
```
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.SGD(lenet.parameters(), lr=0.001, momentum=0.9)
```
最后,我们需要定义一些训练和测试的函数,并开始训练模型。
```
def train(model, dataloader, criterion, optimizer):
model.train()
running_loss = 0.0
correct = 0
total = 0
for i, data in enumerate(dataloader):
inputs, labels = data
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
_, predicted = \
torch.max(outputs.data, dim=1)
total += labels.size(0)
correct += \
(predicted == labels).sum().item()
epoch_loss = running_loss / len(dataloader.dataset)
epoch_acc = correct / total
return epoch_loss, epoch_acc
def test(model, dataloader, criterion):
model.eval()
running_loss = 0.0
correct = 0
total = 0
with torch.no_grad():
for data in dataloader:
inputs, labels = data
outputs = model(inputs)
loss = criterion(outputs, labels)
running_loss += loss.item()
_, predicted = \
torch.max(outputs.data, dim=1)
total += labels.size(0)
correct += \
(predicted == labels).sum().item()
epoch_loss = running_loss / len(dataloader.dataset)
epoch_acc = correct / total
return epoch_loss, epoch_acc
for epoch in range(num_epochs):
train_loss, train_acc = \
train(lenet, train_dataloader, criterion, optimizer)
valid_loss, valid_acc = \
test(lenet, valid_dataloader, criterion)
print(f"Epoch {epoch + 1}: ")
print(f"Train Loss={train_loss:.4f}, Train Acc={train_acc:.4f}")
print(f"Valid Loss={valid_loss:.4f}, Valid Acc={valid_acc:.4f}")
```
此时,我们的模型已经成功训练好了,可以使用测试集进行测试了。测试代码如下:
```
test_loss, test_acc = \
test(lenet, test_dataloader, criterion)
print(f"Test Loss={test_loss:.4f}, Test Acc={test_acc:.4f}")
```
在完成测试后,可以使用以下语句保存该模型:
```
torch.save(lenet.state_dict(), "lenet.pth")
```
上述代码将保存模型的权重参数到文件lenet.pth中。
最后,我们可以使用以下代码加载该模型并对样本进行识别:
```
lenet.load_state_dict(torch.load("lenet.pth"))
lenet.eval()
sample, _ = test_dataset[0]
outputs = lenet(torch.unsqueeze(sample, dim=0))
_, predicted = \
torch.max(outputs.data, dim=1)
print(f"Predicted Label: {predicted.item()}")
```
这段代码将加载保存的模型权重,并使用该模型识别测试集中第一张图片的标签。
### 回答3:
使用pytorch搭建卷积神经网络(Convolutional Neural Network, CNN)识别手写数字,下面是详细步骤:
1. 数据集准备
使用MNIST手写数字数据集,该数据集由60,000个训练图像和10,000个测试图像组成。在pytorch中可以使用torchvision.datasets.MNIST()加载该数据集。
2. 构建CNN模型
使用pytorch的nn.Module来定义CNN模型,其中包括卷积层、ReLU激活函数、池化层以及全连接层等。
3. 定义损失函数和优化器
定义交叉熵损失函数(CrossEntropyLoss)和随机梯度下降优化器(SGD,Stochastic Gradient Descent)。
4. 训练模型
使用dataloader来加载数据集,对模型进行训练,可以使用epoch的方式进行多次训练。
5. 评估模型
在测试集上进行预测,并计算准确率等指标,评估模型的性能。
下面是一份pytorch代码示例:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import torchvision.datasets as datasets
import torchvision.transforms as transforms
# 加载MNIST数据集
transform = transforms.Compose([transforms.ToTensor(),
transforms.Normalize((0.1307,), (0.3081,))])
train_dataset = datasets.MNIST(root='./data', train=True, download=True, transform=transform)
test_dataset = datasets.MNIST(root='./data', train=False, download=True, transform=transform)
batch_size = 32
train_loader = torch.utils.data.DataLoader(train_dataset, batch_size=batch_size, shuffle=True)
test_loader = torch.utils.data.DataLoader(test_dataset, batch_size=batch_size, shuffle=True)
# 构建CNN模型
class CNN(nn.Module):
def __init__(self):
super(CNN, self).__init__()
self.conv1 = nn.Conv2d(in_channels=1, out_channels=32, kernel_size=5, stride=1, padding=2)
self.relu1 = nn.ReLU()
self.pool1 = nn.MaxPool2d(kernel_size=2, stride=2)
self.conv2 = nn.Conv2d(in_channels=32, out_channels=64, kernel_size=5, stride=1, padding=2)
self.relu2 = nn.ReLU()
self.pool2 = nn.MaxPool2d(kernel_size=2, stride=2)
self.fc1 = nn.Linear(7 * 7 * 64, 1024)
self.relu3 = nn.ReLU()
self.fc2 = nn.Linear(1024, 10)
def forward(self, x):
x = self.conv1(x)
x = self.relu1(x)
x = self.pool1(x)
x = self.conv2(x)
x = self.relu2(x)
x = self.pool2(x)
x = x.view(x.size(0), -1)
x = self.fc1(x)
x = self.relu3(x)
x = self.fc2(x)
return x
model = CNN()
print(model)
# 定义损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(model.parameters(), lr=0.01, momentum=0.9)
# 训练模型
num_epochs = 10
for epoch in range(num_epochs):
for i, (images, labels) in enumerate(train_loader):
optimizer.zero_grad()
outputs = model(images)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
if (i+1) % 100 == 0:
print('Epoch [{}/{}], Step [{}/{}], Loss: {:.4f}'
.format(epoch+1, num_epochs, i+1, len(train_loader), loss.item()))
# 评估模型
model.eval()
with torch.no_grad():
correct = 0
total = 0
for images, labels in test_loader:
outputs = model(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print('Accuracy of the network on the 10000 test images: {} %'.format(100 * correct / total))
```
通过训练和评估,我们可以得到一个准确率较高的手写数字识别CNN模型。
相关推荐
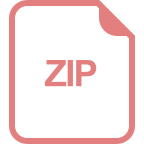
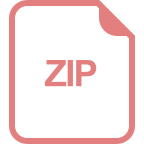
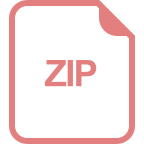

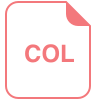
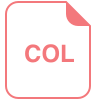
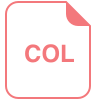
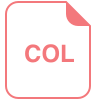







