Unity判断json是否为空
时间: 2023-12-07 14:02:58 浏览: 234
在Unity中,可以使用以下方法来判断一个JSON是否为空:
1. 使用JsonUtility.FromJson方法将JSON字符串转换为对象,然后判断对象是否为null。
```csharp
string jsonString = "{\"name\":\"John\", \"age\":30}";
MyObject myObject = JsonUtility.FromJson<MyObject>(jsonString);
if (myObject == null)
{
Debug.Log("JSON is empty");
}
```
2. 使用JsonUtility.FromJsonOverwrite方法将JSON字符串覆盖到一个已有的对象上,然后判断对象的属性是否为空。
```csharp
string jsonString = "{\"name\":\"John\", \"age\":30}";
MyObject myObject = new MyObject();
JsonUtility.FromJsonOverwrite(jsonString, myObject);
if (string.IsNullOrEmpty(myObject.name) && myObject.age == 0)
{
Debug.Log("JSON is empty");
}
```
3. 使用JsonReader读取JSON字符串,然后判断是否有内容。
```csharp
string jsonString = "{\"name\":\"John\", \"age\":30}";
JsonReader reader = new JsonReader(jsonString);
if (!reader.Read())
{
Debug.Log("JSON is empty");
}
```
以上是三种判断JSON是否为空的方法,可以根据实际情况选择适合自己的方法。
阅读全文
相关推荐
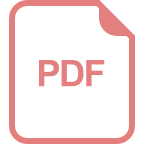
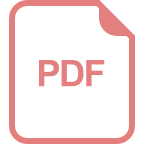


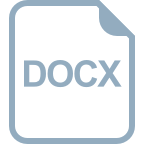
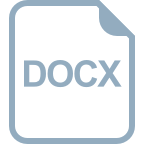


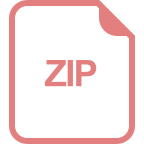
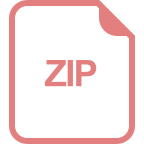
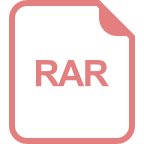
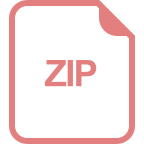
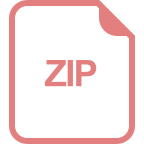
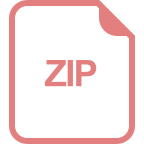