RSA算法Python代码
时间: 2023-11-05 08:00:22 浏览: 91
RSA算法是一种非对称加密算法,它需要一对密钥,一个用于加密,一个用于解密。以下是Python中实现RSA算法的代码:
```python
import random
from math import gcd
def generate_keypair(p: int, q: int) -> tuple:
n = p * q
phi = (p - 1) * (q - 1)
e = random.randrange(1, phi)
g = gcd(e, phi)
while g != 1:
e = random.randrange(1, phi)
g = gcd(e, phi)
d = mod_inverse(e, phi)
return ((e, n), (d, n))
def mod_inverse(a: int, m: int) -> int:
m0 = m
y = 0
x = 1
if m == 1:
return 0
while a > 1:
q = a // m
t = m
m = a % m
a = t
t = y
y = x - q * y
x = t
if x < 0:
x = x + m0
return x
def encrypt(pk: tuple, plaintext: str) -> list:
key, n = pk
cipher = [pow(ord(char), key, n) for char in plaintext]
return cipher
def decrypt(pk: tuple, ciphertext: list) -> str:
key, n = pk
plain = [chr(pow(char, key, n)) for char in ciphertext]
return ''.join(plain)
```
这里的 `generate_keypair` 函数生成了一对公钥和私钥, `encrypt` 函数用公钥加密明文, `decrypt` 函数用私钥解密密文。
阅读全文
相关推荐
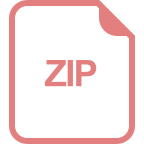


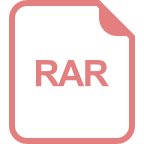
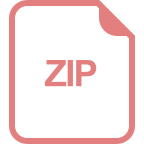








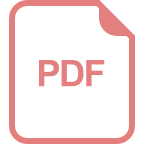

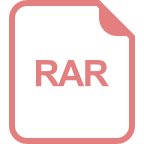