vue3 写滑块验证码
时间: 2023-10-14 15:19:52 浏览: 153
以下是一个简单的滑块验证码的 Vue3 实现:
```vue
<template>
<div class="slider-captcha">
<div class="captcha-container">
<div class="captcha-image" ref="captchaImage">
<img :src="captchaUrl" alt="captcha" @load="handleCaptchaLoad" />
</div>
<div class="slider" ref="slider" @mousedown="handleMouseDown" @mousemove="handleMouseMove" @mouseup="handleMouseUp">
<div class="slider-icon" ref="sliderIcon"></div>
</div>
</div>
<div class="result" v-if="isVerified">验证通过</div>
</div>
</template>
<script>
import { ref } from 'vue';
export default {
name: 'SliderCaptcha',
setup() {
const captchaUrl = ref('https://picsum.photos/300/150');
const isVerified = ref(false);
let isDragging = false;
let startX = 0;
let sliderWidth = 0;
let sliderMaxX = 0;
let dragOffset = 0;
const handleCaptchaLoad = () => {
sliderWidth = Math.floor(getComputedStyle($refs.slider).width.slice(0, -2));
sliderMaxX = sliderWidth - 40;
};
const handleMouseDown = (event) => {
isDragging = true;
startX = event.clientX;
dragOffset = $refs.slider.offsetLeft;
};
const handleMouseMove = (event) => {
if (!isDragging) return;
const x = event.clientX - startX + dragOffset;
$refs.slider.style.left = `${Math.max(0, Math.min(sliderMaxX, x))}px`;
};
const handleMouseUp = () => {
isDragging = false;
if (Math.abs($refs.slider.offsetLeft - sliderMaxX) < 5) {
isVerified.value = true;
} else {
$refs.slider.style.left = '0';
}
};
return {
captchaUrl,
isVerified,
handleCaptchaLoad,
handleMouseDown,
handleMouseMove,
handleMouseUp,
};
},
};
</script>
<style scoped>
.slider-captcha {
position: relative;
width: 300px;
height: 150px;
}
.captcha-container {
position: relative;
width: 100%;
height: 100%;
overflow: hidden;
}
.captcha-image {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
.slider {
position: absolute;
top: 50%;
left: 0;
width: 40px;
height: 40px;
transform: translateY(-50%);
background-color: #ccc;
border-radius: 50%;
cursor: pointer;
}
.slider-icon {
position: absolute;
top: 0;
left: 0;
width: 40px;
height: 40px;
border-radius: 50%;
background-color: #fff;
box-shadow: 1px 1px 3px rgba(0, 0, 0, 0.3);
}
.result {
position: absolute;
top: 50%;
left: 0;
width: 100%;
text-align: center;
font-size: 18px;
color: #fff;
transform: translateY(-50%);
background-color: green;
padding: 10px;
}
</style>
```
这个组件会显示一个随机的图片作为验证码,下面有一个滑块。用户需要拖动滑块,使其到达滑动条的末端,才可通过验证。如果验证通过,会显示一个“验证通过”的提示。
阅读全文
相关推荐
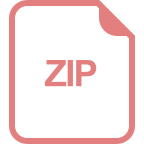

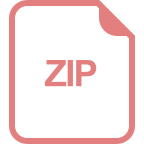
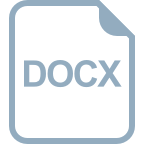
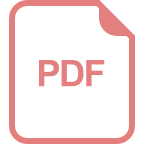
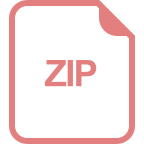
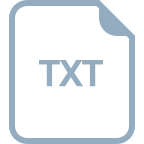
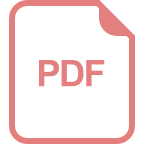
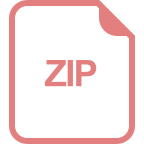
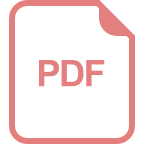
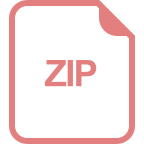
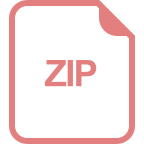
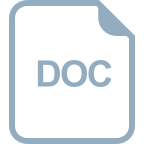
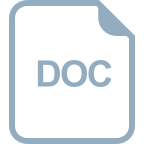

