使用java 编写一个客户端向服务端上传文件的程序,要求使用TCP通信的的知识,完成将本地机器输入的路径下的文件上传到D盘中名称为upload的文件夹中。并把客户端的IP地址加上count标识作为上传后文件的文件名,即IP(count)的形式。其中,count随着文件的增多而增大,例如127.0.0.(1).jpg、127.0.0.(2).jpg。
时间: 2024-03-25 18:40:32 浏览: 58
好的,我可以为您提供一个基本的Java代码示例,用于实现客户端通过TCP通信上传文件到服务器端。请注意,这只是一个示例,并不是生产环境中的最佳实践。
客户端代码:
```java
import java.io.*;
import java.net.*;
public class TCPClient {
public static void main(String[] args) throws Exception {
// 获取客户端IP地址并设置count标识
String ip = InetAddress.getLocalHost().getHostAddress();
int count = 1;
// 建立TCP连接
Socket clientSocket = new Socket("localhost", 8888);
// 获取文件路径
String filePath = "C:/path/to/file.ext";
// 读取文件内容
File file = new File(filePath);
byte[] fileContent = new byte[(int) file.length()];
FileInputStream fis = new FileInputStream(file);
fis.read(fileContent);
fis.close();
// 发送文件名和内容
OutputStream os = clientSocket.getOutputStream();
String fileName = "upload/" + ip + "(" + count + ")" + file.getName();
os.write(fileName.getBytes());
os.write(fileContent);
os.flush();
os.close();
// 关闭TCP连接
clientSocket.close();
}
}
```
服务器端代码:
```java
import java.io.*;
import java.net.*;
public class TCPServer {
public static void main(String[] args) throws Exception {
// 建立TCP监听
ServerSocket serverSocket = new ServerSocket(8888);
while (true) {
// 接收TCP连接
Socket connectionSocket = serverSocket.accept();
// 读取文件名和内容
InputStream is = connectionSocket.getInputStream();
byte[] fileNameBytes = new byte[1024];
int bytesRead = is.read(fileNameBytes);
String fileName = new String(fileNameBytes, 0, bytesRead);
byte[] fileContent = new byte[1024];
ByteArrayOutputStream baos = new ByteArrayOutputStream();
while ((bytesRead = is.read(fileContent)) != -1) {
baos.write(fileContent, 0, bytesRead);
}
byte[] content = baos.toByteArray();
baos.close();
// 写入文件
File file = new File("D:/" + fileName);
FileOutputStream fos = new FileOutputStream(file);
fos.write(content);
fos.flush();
fos.close();
// 关闭TCP连接
connectionSocket.close();
}
}
}
```
请注意,这只是一个简单的示例,需要您根据实际需求进行修改和完善。例如,您可能需要添加错误处理和日志记录等功能,以提高程序的稳定性和可靠性。
阅读全文
相关推荐
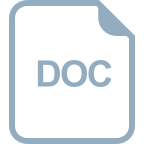
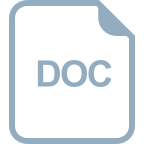
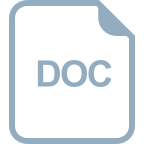
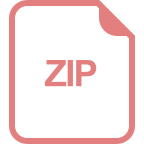
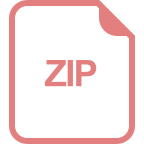
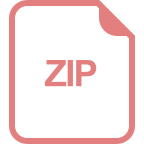
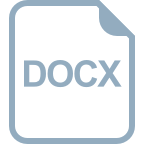
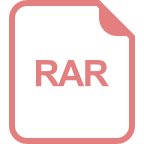
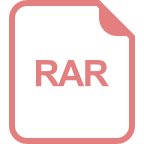
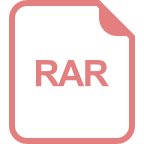
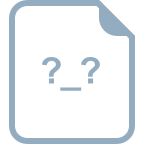
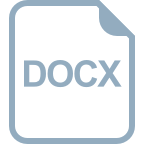
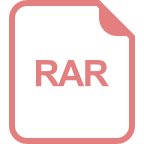
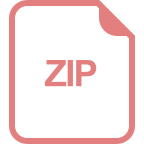
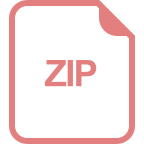
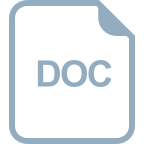
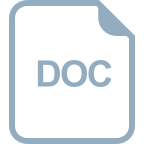
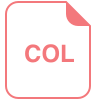
