利用libubox中的uloop机制,写一个应用,监听设备节点 "/run/ql_pcmv_pipe_rx"是否存在可读数据,存在可读数据,将数据透传到另一个设备节点 "/dev/mhi_pipe_68"
时间: 2024-09-09 10:02:07 浏览: 48
利用libuavcan中的uloop机制编写一个Linux C程序来监控设备节点"/run/ql_pcmv_pipe_rx",当有可读数据时,可以将这些数据转发到"/dev/mhi_pipe_68",可以按照以下步骤来实现:
首先,你需要包含必要的头文件并初始化uloop循环:
```c
#include <stdio.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/stat.h>
#include <linux/fs.h>
#include <linux/uinput.h>
#include "ulib.h"
static int pipe_fd_in = -1;
static int pipe_fd_out = -1;
void *listener_thread(void *data) {
struct pollfd fds[2];
int ret;
if ((pipe_fd_in = open("/run/ql_pcmv_pipe_rx", O_RDONLY | O_NONBLOCK)) < 0) {
perror("Failed to open input pipe");
return NULL;
}
if ((pipe_fd_out = open("/dev/mhi_pipe_68", O_WRONLY)) < 0) {
perror("Failed to open output pipe");
close(pipe_fd_in);
return NULL;
}
fds[0].fd = pipe_fd_in;
fds[0].events = POLLIN;
fds[1].fd = pipe_fd_out;
fds[1].events = POLLOUT;
while (true) {
ret = poll(fds, 2, -1);
if (ret == -1 && errno != EINTR) {
perror("poll error");
break;
}
if (fds[0].revents & POLLIN) { // Data available on input pipe
ssize_t read_size = read(pipe_fd_in, /* buffer here... */, sizeof(buffer));
if (read_size > 0) {
write(pipe_fd_out, /* data from buffer here... */, read_size);
printf("Data transferred from /run/ql_pcmv_pipe_rx to /dev/mhi_pipe_68\n");
} else if (read_size == 0) { // Pipe closed by other end
perror("Pipe EOF detected");
break;
} else {
perror("Error reading from input pipe");
}
}
if (fds[1].revents & POLLOUT && /* buffer is not empty or some error occurred */ ) {
/* Handle any pending write operations */
}
}
close(pipe_fd_in);
close(pipe_fd_out);
return NULL;
}
```
然后你可以创建一个uloop实例,并添加监听任务到线程中:
```c
ULoop *loop;
void *thread_id;
int main() {
loop = uloop_new();
thread_id = uloop_thread_start(listener_thread, NULL, ULOOP_THREAD_SAFE);
uloop_run();
uloop_thread_stop(thread_id);
uloop_unref(loop);
return 0;
}
```
注意:这个例子假设`buffer`已声明并足够大以容纳从输入管道读取的数据。此外,为了优雅地处理错误和关闭条件,你可能需要加入更多的异常处理代码。同时,确保权限允许应用程序读写指定的设备。
阅读全文
相关推荐
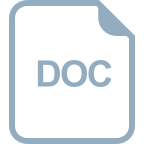
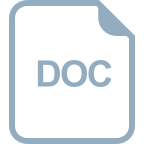
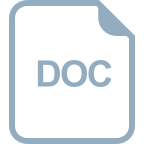
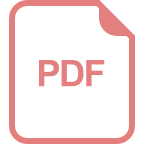
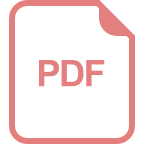
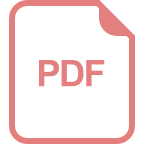
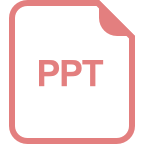
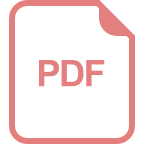
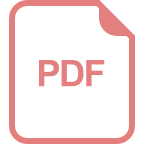
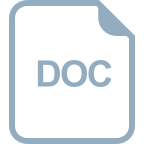
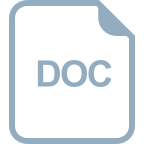