把其他的功能代码也实现
时间: 2024-10-13 10:10:34 浏览: 8
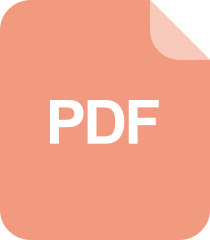
Android倒计时功能的实现代码
除了上述提到的基本功能之外,为了完善通讯录管理系统,我们还需要实现修改联系人和清空联系人的功能。以下是完整代码示例:
```cpp
#include <iostream>
#include <map>
#include <string>
#include <algorithm> // 使用这个头文件用于std::find
// 保持前面的Contact结构体不变
class AddressBook {
private:
std::map<std::string, Contact> contacts;
public:
// 菜单功能项
void displayMenu() {
std::cout << "1. 添加联系人\n"
<< "2. 显示联系人\n"
<< "3. 删除联系人\n"
<< "4. 查找联系人\n"
<< "5. 修改联系人\n"
<< "6. 清空联系人\n"
<< "7. 退出\n";
}
// 添加联系人
// ...
// 显示联系人
// ...
// 删除联系人
// 已有实现,无需改变
// 查找联系人
const Contact* findContact(const std::string& name) const {
auto it = contacts.find(name);
return it == contacts.end() ? nullptr : &it->second;
}
// 修改联系人
bool modifyContact(const std::string& oldName, const Contact& updatedContact) {
auto it = contacts.find(oldName);
if (it != contacts.end()) {
it->second = updatedContact;
std::cout << "联系人信息已更新。\n";
return true;
}
std::cout << "未找到该联系人。\n";
return false;
}
// 清空联系人
void clearContacts() {
contacts.clear();
std::cout << "联系人已清空。\n";
}
// 主循环
void run() {
while (true) {
displayMenu();
int choice;
std::cin >> choice;
switch (choice) {
// ... 其他已有的case处理
case 5: // 修改联系人
std::string oldName, newName;
std::cin >> oldName >> newName;
if (!oldName.empty() && !newName.empty()) {
Contact newContact;
std::cout << "请输入新联系人的信息:" << std::endl;
// 获取新的联系人信息并调用modifyContact
// 提供用户输入并验证数据的函数
// ...
} else {
std::cout << "请输入完整的姓名。\n";
}
break;
case 6: // 清空联系人
clearContacts();
break;
default:
// ... 其他case处理
}
}
}
};
int main() {
AddressBook book;
book.run();
return 0;
}
```
在这个版本里,我们添加了`modifyContact`函数来实现修改联系人功能,以及在`run`函数内部处理用户输入并调用相应的方法。
阅读全文
相关推荐
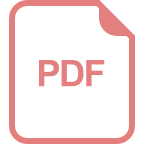
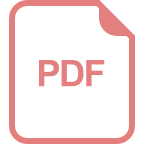
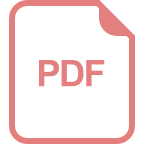
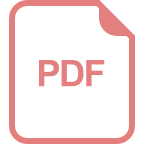
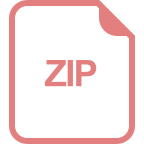
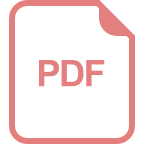
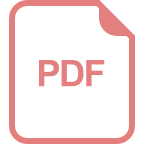
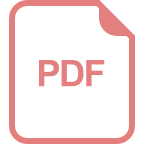
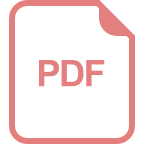
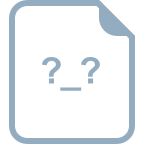