python 读取图像文件列表
时间: 2023-11-14 19:11:03 浏览: 101
Python可以使用OpenCV库来读取图像文件列表。以下是一个简单的示例代码:
```python
import cv2
import os
# 图像文件夹路径
img_folder = 'path/to/folder'
# 获取文件夹中所有图像文件的路径
img_paths = [os.path.join(img_folder, f) for f in os.listdir(img_folder) if f.endswith('.jpg')]
# 读取每个图像文件并将其转换为矩阵
img_matrices = []
for img_path in img_paths:
img = cv2.imread(img_path)
img_matrices.append(img)
```
上述代码中,首先定义了一个图像文件夹的路径,然后使用`os.listdir`函数获取该文件夹中所有以`.jpg`结尾的文件的路径。接下来,使用OpenCV的`cv2.imread`函数读取每个图像文件,并将其转换为矩阵。最后,将所有矩阵存储在一个列表中。
相关问题
如何使用Python读取图像文件?
使用Python读取图像文件可以使用第三方库PIL(Python Imaging Library)或者OpenCV库。下面是两种方法的示例:
1. 使用PIL库读取图像文件:
```python
from PIL import Image
# 打开图像文件
image = Image.open("image.jpg")
# 获取图像的宽度和高度
width, height = image.size
# 显示图像
image.show()
# 关闭图像文件
image.close()
```
2. 使用OpenCV库读取图像文件:
```python
import cv2
# 读取图像文件
image = cv2.imread("image.jpg")
# 获取图像的宽度和高度
height, width, channels = image.shape
# 显示图像
cv2.imshow("Image", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
python 读取图片为数字矩阵_python读取图像矩阵文件并转换为向量实例
读取图片为数字矩阵的代码示例:
```python
from PIL import Image
# 读取图片
img = Image.open('example.jpg')
# 转换为灰度图
gray_img = img.convert('L')
# 转换为矩阵
matrix = list(gray_img.getdata())
# 打印矩阵
print(matrix)
```
将图像矩阵文件转换为向量的代码示例:
```python
import numpy as np
# 读取矩阵文件
matrix = np.loadtxt('matrix.txt')
# 将矩阵转换为向量
vector = matrix.reshape(-1)
# 打印向量
print(vector)
```
其中,`example.jpg` 是要读取的图片文件名,`matrix.txt` 是要读取的图像矩阵文件名。
阅读全文
相关推荐
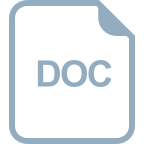
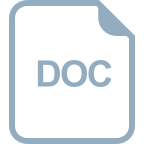
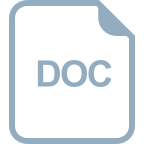
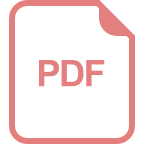
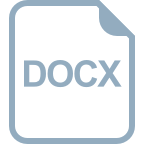
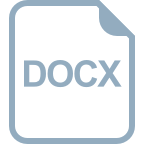
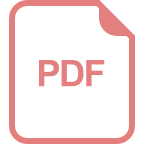
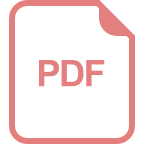
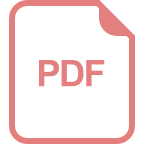






