fruits=['strawberry','fig','apple','cherry','raspberry','banana'] print(sorted(fruits,key=len)) # 输出结果如下: # ['fig', 'apple', 'cherry', 'banana', 'raspberry', 'strawberry']
时间: 2023-08-20 12:06:18 浏览: 186
你的代码是正确的。`sorted()` 函数可以按照指定的排序规则对列表进行排序。
在你的代码中,`key=len` 表示使用元素的长度作为排序的依据。因此,`sorted(fruits, key=len)` 将列表 `fruits` 按照元素的长度进行升序排序。
输出结果为 `['fig', 'apple', 'cherry', 'banana', 'raspberry', 'strawberry']`,符合你所期望的结果。
如果还有其他问题,请随时提问。
相关问题
fruits = ["banana","apple","pear","watermelon"] print(sorted(fruits, key = len)[2])
这段代码的输出结果为 "pear"。
首先,我们将列表 fruits 传递给 sorted 函数,并通过关键字参数 key=len 指定使用元素的长度作为排序的依据。
这将返回一个新的已排序的列表,即 ["pear", "apple", "banana", "watermelon"]。最后,我们使用索引值 [2] 来访问这个列表中的第三个元素,即 "pear"。注意,索引值从 0 开始计数。
fruits = ["apple", "banana", "cherry"] python中 将fruits中的 "apple"换成"origin"
### 如何在Python中替换列表中的元素
在Python中,可以通过多种方式来实现列表中特定元素的替换操作。对于给定的任务——将列表`fruits`中的字符串`'apple'`替换为`'origin'`,可以采用如下所示的方法:
#### 方法一:通过遍历并修改
可以直接遍历列表找到目标元素的位置,并将其替换成新的值。
```python
fruits = ['banana', 'apple', 'cherry']
for index, fruit in enumerate(fruits):
if fruit == 'apple':
fruits[index] = 'origin'
print(fruits) # 输出: ['banana', 'origin', 'cherry']
```
这种方法利用了`enumerate()`函数获取索引及其对应的元素[^1]。
#### 方法二:使用列表推导式
如果希望创建一个新的列表而不是原地修改旧列表,则可以考虑使用列表推导式的简洁语法完成此任务。
```python
fruits = ['banana', 'apple', 'cherry']
new_fruits = ['origin' if item == 'apple' else item for item in fruits]
print(new_fruits) # 输出: ['banana', 'origin', 'cherry']
```
这种方式不仅能够有效地处理单次匹配的情况,还可以轻松扩展到多条件判断场景下。
#### 方法三:使用内置方法replace(针对字符串)
需要注意的是,上述两种方法适用于任何类型的可变序列。然而,在某些特殊情况下,比如当列表只包含字符串时,也可以尝试先转换成字符串再做整体替换最后重新分割回列表的方式,但这通常不是最推荐的做法因为效率较低而且容易引入额外复杂度。
阅读全文
相关推荐
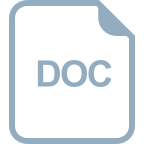















