python class装饰器
时间: 2023-11-13 11:14:36 浏览: 86
Python中的装饰器是一种特殊的语法,用于修改或增强函数或类的行为。装饰器本质上是一个函数(或类),它接受一个函数(或类)作为参数,并返回一个新的函数(或类)。
对于类装饰器,它们接受一个类作为参数,并返回一个修改后的类。以下是一个示例:
```python
def decorator(cls):
# 在这里可以对类进行修改或增强
# 在这个示例中,我们给类添加了一个新的属性和一个新的方法
cls.new_property = "This is a new property"
def new_method(self):
return "This is a new method"
cls.new_method = new_method
return cls
@decorator
class MyClass:
def __init__(self):
self.property = "This is the original property"
def original_method(self):
return "This is the original method"
```
在上面的示例中,`decorator` 函数接受 `MyClass` 类作为参数,并在其中添加了一个新的属性 `new_property` 和一个新的方法 `new_method`。通过将 `decorator` 函数应用于 `MyClass` 类,我们修改了原始的 `MyClass` 类,使其具有了新的属性和方法。
现在,我们可以实例化 `MyClass` 并访问它的属性和方法:
```python
obj = MyClass()
print(obj.property) # 输出: This is the original property
print(obj.new_property) # 输出: This is a new property
print(obj.original_method()) # 输出: This is the original method
print(obj.new_method()) # 输出: This is a new method
```
通过类装饰器,我们可以在不修改原始类定义的情况下,动态地增加新的功能或属性。这是Python中非常强大和灵活的特性之一。
阅读全文
相关推荐
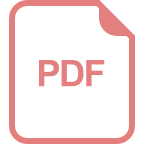
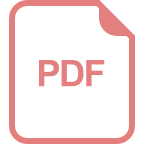
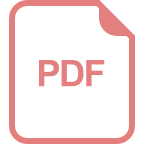













