如何在Python中创建一个加减乘除的数学运算函数库,并使用文档字符串详细说明每个函数的功能?
时间: 2024-11-05 12:13:02 浏览: 30
要创建一个包含加减乘除功能的数学运算函数库,你可以使用Python中的函数定义语法,并为每个函数添加文档字符串以说明其用途。以下是一个基本的指导,它涵盖了如何定义这些函数,并展示了如何为它们添加文档字符串。建议参考《Python函数入门指南:定义与示例》来获得更深入的理解和实践。
参考资源链接:[Python函数入门指南:定义与示例](https://wenku.csdn.net/doc/4a4ehb9ym2?spm=1055.2569.3001.10343)
首先,我们需要定义每个运算函数,如加法、减法、乘法和除法。每个函数都将接受两个参数,并返回相应的运算结果。在此基础上,我们可以加入异常处理来确保程序的健壮性,例如在除法中防止除以零的错误。
以下是一个示例代码片段,展示了如何定义这些函数:
```python
def add(a, b):
参考资源链接:[Python函数入门指南:定义与示例](https://wenku.csdn.net/doc/4a4ehb9ym2?spm=1055.2569.3001.10343)
相关问题
附件里是一个计算24点的小程序,它的原理是在1~9之间随机生成4个数,他们经过加减乘除运算一定能等于24(不考虑带括号的情况),然后把这些数显示在GUI界面,用户可以通过键盘的光标键改变运算符使等式成立。 使用Python GUI编程实现该程序。 提示:使用eval()函数可以把字符串形式的数学表达式变成数值形式的表达式。如print(eval("3+2*5")),则打印出13。
好的,我会为您详细解答这个问题。
首先,您需要安装Python的GUI编程库,比如tkinter或PyQt5。这里我们以tkinter为例。
接下来,您可以创建一个窗口,并在窗口中添加4个随机生成的数字和4个按钮,分别表示加减乘除四种运算符。
然后,您需要编写一个函数来计算用户输入的表达式是否等于24。可以使用eval()函数将用户输入的字符串表达式计算出结果,然后与24进行比较。
最后,您需要将用户输入的表达式显示在GUI界面上,并提示用户是否等于24。
下面是一个简单的示例代码,仅供参考:
```python
import tkinter as tk
import random
# 生成四个随机数
numbers = [random.randint(1, 9) for i in range(4)]
# 计算表达式是否等于24
def calculate():
expression = ''.join(str(numbers[i]) + operator[i] for i in range(4)) + str(numbers[3])
result = eval(expression)
if result == 24:
result_label.config(text='Congratulations! You got it!')
else:
result_label.config(text='Sorry, try again.')
# 更新运算符
def update_operator(index, op):
operator[index] = op
# 创建窗口和组件
root = tk.Tk()
root.title('24-Game')
num1_label = tk.Label(root, text=numbers[0])
num2_label = tk.Label(root, text=numbers[1])
num3_label = tk.Label(root, text=numbers[2])
num4_label = tk.Label(root, text=numbers[3])
plus_button = tk.Button(root, text='+', command=lambda: update_operator(0, '+'))
minus_button = tk.Button(root, text='-', command=lambda: update_operator(0, '-'))
multiply_button = tk.Button(root, text='*', command=lambda: update_operator(0, '*'))
divide_button = tk.Button(root, text='/', command=lambda: update_operator(0, '/'))
plus_button2 = tk.Button(root, text='+', command=lambda: update_operator(1, '+'))
minus_button2 = tk.Button(root, text='-', command=lambda: update_operator(1, '-'))
multiply_button2 = tk.Button(root, text='*', command=lambda: update_operator(1, '*'))
divide_button2 = tk.Button(root, text='/', command=lambda: update_operator(1, '/'))
plus_button3 = tk.Button(root, text='+', command=lambda: update_operator(2, '+'))
minus_button3 = tk.Button(root, text='-', command=lambda: update_operator(2, '-'))
multiply_button3 = tk.Button(root, text='*', command=lambda: update_operator(2, '*'))
divide_button3 = tk.Button(root, text='/', command=lambda: update_operator(2, '/'))
result_label = tk.Label(root, text='')
calculate_button = tk.Button(root, text='Calculate', command=calculate)
# 放置组件
num1_label.grid(row=0, column=0)
num2_label.grid(row=0, column=1)
num3_label.grid(row=0, column=2)
num4_label.grid(row=0, column=3)
plus_button.grid(row=1, column=0)
minus_button.grid(row=2, column=0)
multiply_button.grid(row=3, column=0)
divide_button.grid(row=4, column=0)
plus_button2.grid(row=1, column=1)
minus_button2.grid(row=2, column=1)
multiply_button2.grid(row=3, column=1)
divide_button2.grid(row=4, column=1)
plus_button3.grid(row=1, column=2)
minus_button3.grid(row=2, column=2)
multiply_button3.grid(row=3, column=2)
divide_button3.grid(row=4, column=2)
calculate_button.grid(row=5, column=0, columnspan=4)
result_label.grid(row=6, column=0, columnspan=4)
operator = ['+', '+', '+']
root.mainloop()
```
斐波那契数列,从键盘接收1个,或者若干个字符串,将其改为整形数字,进行加减乘除乘方开方等运算,显示结果
斐波那契数列是一个经典的数学序列,它的特点是每个数都是前两个数之和,通常用0和1开始。例如,前几个数字是0, 1, 1, 2, 3, 5...。如果你需要用户输入一串包含斐波那契数的字符串,并将它们转换成整数进行算术运算,可以按照以下步骤操作:
1. **输入处理**:
- 首先,从键盘接收用户的输入,这可能是一系列由空格分隔的数字字符串。
2. **字符串转整数**:
- 对于每个接收到的字符串,使用`int()`函数尝试转换它,如果输入是可以转换成功的数字,就得到对应的整数值;否则,可能需要处理错误情况,如提示用户输入无效。
3. **计算**:
- 将用户输入的整数进行加减乘除乘方或者开方运算,比如`a + b`, `a * b`, `math.pow(a, b)`等,其中`math`表示Python中的数学库。
4. **结果显示**:
- 计算完成后,将结果显示给用户。如果涉及多个操作,可以分别显示结果,或者组合所有的计算一起显示。
5. **异常处理**:
- 进行必要的错误检查,比如除法运算可能会遇到除数为零的情况,开方可能处理负数等。
举例代码(Python):
```python
import math
# 接收用户输入并分割
input_str = input("请输入斐波那契数列表,用空格隔开:")
numbers = [int(num) for num in input_str.split()]
# 对每个数进行运算
result = sum(numbers)
for i in range(len(numbers)):
if i < len(numbers) - 1:
result += numbers[i] * numbers[i+1]
else:
break
try:
result_squared = math.sqrt(result)
except ValueError:
print(f"开方运算失败,因为结果不是非负数:{result}")
print(f"计算结果:{result},平方根结果(若有效):{result_squared}")
```
阅读全文
相关推荐
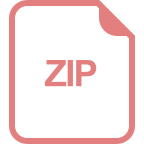
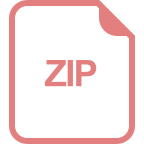
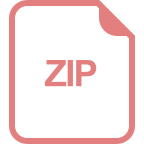
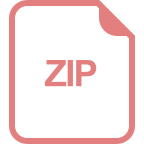
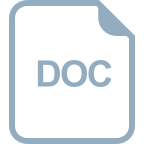
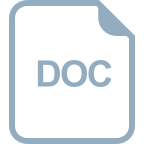
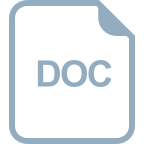
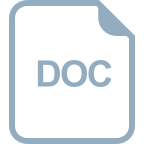
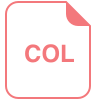







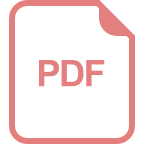