重写copyProperties
时间: 2023-08-19 13:13:02 浏览: 47
当你说"重写copyProperties",我假设你是想要自定义一个方法来复制对象属性。在Java中,你可以使用反射机制来实现类似的功能。下面是一个示例代码,展示了如何使用反射来复制对象属性:
```java
import java.lang.reflect.Field;
public class CopyUtils {
public static void copyProperties(Object source, Object destination) {
if (source == null || destination == null) {
throw new IllegalArgumentException("Source and destination objects must not be null");
}
Class<?> sourceClass = source.getClass();
Class<?> destinationClass = destination.getClass();
Field[] sourceFields = sourceClass.getDeclaredFields();
Field[] destinationFields = destinationClass.getDeclaredFields();
for (Field sourceField : sourceFields) {
for (Field destinationField : destinationFields) {
if (sourceField.getName().equals(destinationField.getName())
&& sourceField.getType().equals(destinationField.getType())) {
try {
sourceField.setAccessible(true);
destinationField.setAccessible(true);
Object value = sourceField.get(source);
destinationField.set(destination, value);
} catch (IllegalAccessException e) {
e.printStackTrace();
}
break;
}
}
}
}
}
```
你可以使用上述的 `copyProperties` 方法来复制两个对象的属性。例如:
```java
class Person {
private String name;
private int age;
// getters and setters...
}
Person source = new Person();
source.setName("John");
source.setAge(30);
Person destination = new Person();
CopyUtils.copyProperties(source, destination);
System.out.println(destination.getName()); // 输出: John
System.out.println(destination.getAge()); // 输出: 30
```
希望这个示例对你有帮助!如果你有其他问题,请随时提问。
相关推荐
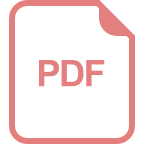
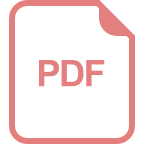
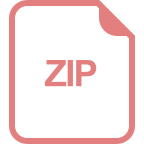













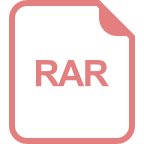
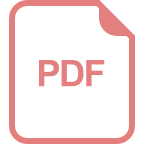