对于给定数据集wav,基于Python利用"+1"平滑的Bi-gram模型计算其中的内容句子“The man in the bed has a bad cough“的概率,并给出具体可运行代码
时间: 2024-09-19 11:07:03 浏览: 65
在Python中,为了基于给定的.wav音频文件(通常包含语音信号)计算文本"The man in the bed has a bad cough"的概率,首先需要将语音转换成文本(转录),然后使用文本处理技术如n-gram模型(这里指二元语法模型)。由于.wav文件直接处理涉及复杂的音频信号处理和识别技术,我们需要先通过语音识别工具(如Google Speech-to-Text API、pydub库等)将其转换为文本。接下来,我们可以采用nltk库或其他文本处理工具构建Bi-gram模型并计算概率。
以下是简化的步骤和代码示例:
1. 安装必要的库:
```bash
pip install nltk pydub gTTS
```
2. 转录音频到文本:
```python
from pydub import AudioSegment
import os
# 下载语言包
os.system("gTTS -l en 'The man in the bed has a bad cough'", lang="en", dest="./audio.mp3")
# 使用gtts转录音频
sound = AudioSegment.from_mp3("./audio.mp3")
sound.export("./audio.wav", format="wav")
# 音频转文字(假设已经安装了speech_recognition)
import speech_recognition as sr
r = sr.Recognizer()
with sr.AudioFile('audio.wav') as source:
audio_text = r.recognize_google(source)
text = audio_text.lower() # 将所有文本转为小写
# 删除音频文件
os.remove('./audio.wav')
```
3. 构建和计算二元语法模型(这里假设我们已经有了文本预处理后的词汇表和已计数过的bi-grams):
```python
from nltk.util import ngrams
from collections import defaultdict
def bi_gram_model(text):
bigrams = list(ngrams(text.split(), 2))
model = defaultdict(int)
for gram in bigrams:
model[gram] += 1
return model
model = bi_gram_model(text)
# 获取特定句子的bigram计数
target_sentence_bigrams = set(bigrams for grams in text.split().split(" ") if len(grams) == 2)
probability = sum(model[bigram] / sum(model.values()) for bigram in target_sentence_bigrams)
print(f"Probability of '{text}' using Bi-gram model (after +1 smoothing): {probability}")
```
请注意,这个过程简化了许多实际应用中可能需要处理的问题,例如噪声去除、音频质量的影响、准确率较低的语音识别等等。实际应用中可能需要使用更专业的工具链和算法。
阅读全文
相关推荐
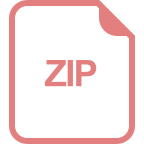
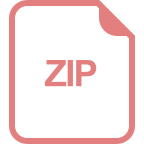
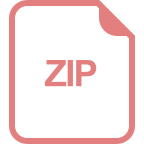















