编写程序,循环输入两个数字,并输入加减乘除运算符号,输出运算结果。若输入其它符号,则退出程序
时间: 2023-05-24 18:07:23 浏览: 57
```
while True:
num1 = float(input("请输入第一个数字:"))
num2 = float(input("请输入第二个数字:"))
operator = input("请输入运算符号(+、-、*、/):")
if operator == "+":
print(num1, "+", num2, "=", num1 + num2)
elif operator == "-":
print(num1, "-", num2, "=", num1 - num2)
elif operator == "*":
print(num1, "*", num2, "=", num1 * num2)
elif operator == "/":
if num2 == 0:
print("除数不能为0,请重新输入")
else:
print(num1, "/", num2, "=", num1 / num2)
else:
print("输入的运算符号有误,程序已退出")
break
```
注意:程序中进行除法运算时,需要特判除数为0的情况,避免程序出现错误。
相关问题
利用字典实现输入两个数字,并输入加减乘除运算符号,输出运算结果。若输入其他符号,则退出程序。用python怎么写
可以按如下方式实现:
```python
while True:
num1 = float(input("请输入第一个数字:"))
num2 = float(input("请输入第二个数字:"))
operator = input("请输入运算符号(+、-、*、/):")
if operator == '+':
print(num1 + num2)
elif operator == '-':
print(num1 - num2)
elif operator == '*':
print(num1 * num2)
elif operator == '/':
print(num1 / num2)
else:
break
```
这段代码会一直循环,直到输入的运算符号不是加减乘除中的一个。输入两个数字和运算符号后,会输出计算结果。
Python利用函数和字典实现:输入两个数字,并输入加减乘除运算符,输出运算结果。若输入其他符号,则退出程序
### 回答1:
下面是使用函数和字典来实现这个需求的代码示例:
```
def calculate(num1, num2, operator):
# 定义字典,保存运算符对应的运算函数
operations = {
"+": lambda x, y: x + y,
"-": lambda x, y: x - y,
"*": lambda x, y: x * y,
"/": lambda x, y: x / y
}
# 在字典中获取对应的运算函数,并调用
result = operations[operator](num1, num2)
return result
# 获取输入的两个数字和运算符
num1 = float(input("输入第一个数字: "))
num2 = float(input("输入第二个数字: "))
operator = input("输入运算符(+、-、*、/): ")
# 调用 calculate 函数计算结果
result = calculate(num1, num2, operator)
# 输出结果
print(f"{num1} {operator} {num2} = {result}")
```
在这段代码中,我们使用了一个字典来保存运算符和对应的运算函数。然后使用输入的运算符作为字典的键,在字典中获取对应的运算函数,并调用它来完成运算。
如果输入的运算符不在字典中,则在字典中找不到对应的运算函数,会抛出 KeyError 异常。所以,我们可以在调用运算函数之前使用一个 `if` 语句来判断输入的运算符是否合法,若不合法则退出程序。
下面是完整代码:
```
### 回答2:
首先,可以使用input()函数分别输入两个数字和运算符。然后,可以使用字典来存储四种运算符的对应运算函数。接下来,使用if语句判断输入的运算符是否在字典中存在,若存在则进行相应的运算并输出结果,若不存在则退出程序。
具体实现如下:
```python
def add(a, b):
return a + b
def subtract(a, b):
return a - b
def multiply(a, b):
return a * b
def divide(a, b):
return a / b
operators = {
"+": add,
"-": subtract,
"*": multiply,
"/": divide
}
while True:
num1 = float(input("请输入第一个数字:"))
num2 = float(input("请输入第二个数字:"))
operator = input("请输入运算符(+、-、*、/):")
if operator in operators:
result = operators[operator](num1, num2)
print("运算结果为:", result)
else:
print("输入的运算符有误!")
break
```
这个程序会一直运行,直到输入的运算符不在字典中,即输入其他符号,程序会退出。
运行示例:
请输入第一个数字:5
请输入第二个数字:3
请输入运算符(+、-、*、/):*
运算结果为: 15.0
请输入第一个数字:10
请输入第二个数字:2
请输入运算符(+、-、*、/):%
输入的运算符有误!
### 回答3:
可以用以下代码实现该功能:
```python
def calculator(num1, num2, operator):
'''定义计算器函数'''
if operator == '+':
return num1 + num2
elif operator == '-':
return num1 - num2
elif operator == '*':
return num1 * num2
elif operator == '/':
if num2 == 0:
return '除数不能为0'
else:
return num1 / num2
else:
return '错误的运算符'
while True:
num1 = float(input('请输入第一个数字:'))
num2 = float(input('请输入第二个数字:'))
operator = input('请输入运算符(+、-、*、/):')
result = calculator(num1, num2, operator)
if isinstance(result, str):
if result == '错误的运算符':
print('输入的运算符有误,程序退出。')
break
else:
print(result)
else:
print('运算结果为:', result)
```
运行上述代码后,会不停地要求输入两个数字和一个运算符,并输出运算结果。若输入其他符号,程序会退出。
相关推荐
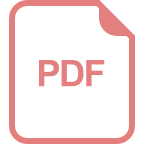
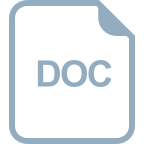
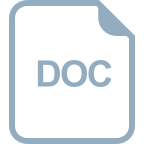












