import json import subprocess with open('/var/log/suricata/fast.log', 'r') as f: events = json.load(f) for event in events: if event['alert']['severity'] <= 2: ip = event['src_ip'] # 调用iptables命令封禁IP result = subprocess.call(['iptables', '-A', 'INPUT', '-s', ip, '-j', 'DROP']) if result == 0: print(f'封禁IP {ip} 成功!') else: print(f'封禁IP {ip} 失败!') 代码运行后报错:Traceback (most recent call last): File "do-Suricata.py", line 6, in <module> event = json.loads(line) File "/usr/local/python3.7/lib/python3.7/json/__init__.py", line 348, in loads return _default_decoder.decode(s) File "/usr/local/python3.7/lib/python3.7/json/decoder.py", line 340, in decode raise JSONDecodeError("Extra data", s, end) json.decoder.JSONDecodeError: Extra data: line 1 column 2 (char 1)如何解决
时间: 2023-12-03 17:47:14 浏览: 109
这个错误提示说明在读取 `/var/log/suricata/fast.log` 文件时,有一些额外的数据没有被解析掉,导致 `json.loads` 函数无法正常工作。你需要检查一下文件内容,看看是否存在格式错误或者其他问题。
另外,你的代码中使用了 `json.load` 函数,这个函数是一次性读取整个 JSON 文件内容并解析成 Python 对象,可能会导致内存占用过大。建议改为逐行读取文件并解析每一行的 JSON 字符串,例如:
```
import json
import subprocess
with open('/var/log/suricata/fast.log', 'r') as f:
for line in f:
try:
event = json.loads(line)
except json.JSONDecodeError:
# 如果解析失败,则跳过这一行
continue
if event['alert']['severity'] <= 2:
ip = event['src_ip']
# 调用iptables命令封禁IP
result = subprocess.call(['iptables', '-A', 'INPUT', '-s', ip, '-j', 'DROP'])
if result == 0:
print(f'封禁IP {ip} 成功!')
else:
print(f'封禁IP {ip} 失败!')
```
这样做可以避免一次性读取整个文件,减小内存占用。同时,也可以更好地处理文件中可能存在的格式错误或其他异常情况。
阅读全文
相关推荐
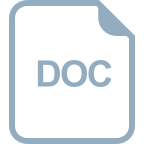
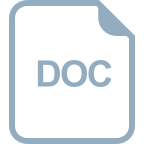
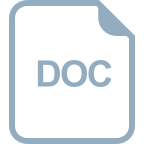










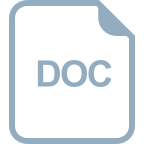
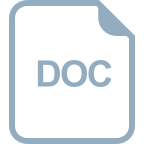