class Tree { constructor(value) { this.value = value; this.children = []; } addChild(value) { this.children.push(new Tree(value)); } inorder() { let result = []; for (let child of this.children) { result = result.concat(child.inorder()); } return result.concat(this.value); } preorder() { let result = [this.value]; for (let child of this.children) { result = result.concat(child.preorder()); } return result; } postorder() { let result = []; for (let child of this.children) { result = result.concat(child.postorder()); } return result.concat(this.value); } modifyValue(newValue) { this.value = newValue; } findNode(value) { if (this.value === value) return this; for (let child of this.children) { const result = child.findNode(value); if (result) return result; } return null; } }请给我写出他的调试代码
时间: 2024-03-25 17:35:59 浏览: 59
以下是一个简单的调试代码,用于测试 Tree 类的一些基本功能:
```javascript
const tree = new Tree(1);
tree.addChild(2);
tree.addChild(3);
tree.children[0].addChild(4);
tree.children[1].addChild(5);
tree.children[1].addChild(6);
console.log(tree.inorder()); // 输出 [4, 2, 1, 5, 6, 3]
console.log(tree.preorder()); // 输出 [1, 2, 4, 3, 5, 6]
console.log(tree.postorder()); // 输出 [4, 2, 5, 6, 3, 1]
const node = tree.findNode(4);
console.log(node); // 输出 Tree { value: 4, children: [] }
tree.modifyValue(7);
console.log(tree.value); // 输出 7
```
在这个例子中,我们首先创建了一个包含 6 个节点的树,然后测试了它的 inorder、preorder 和 postorder 遍历方法。接下来,我们使用 findNode 方法查找值为 4 的节点,并测试了 modifyValue 方法修改树的根节点的值。
相关问题
class Tree{ constructor(value){ this.value=value; this.children=[]; } addChild(value){ this.child.push(new Tree(value)); } inorder(){ if(this.children.length)return[this.value]; let result=[]; for(let child of this.children){ result=result.concat(child.inorder());} return[this.value].concat(result); } preorder(){ let result=[this.value]; for(let child of this.children){ result=result.concat(child.preorder()); } return result; } postorder(){ if(!this.children.length)return[this.value]; let result=[]; for(let child of this.children){ result=result.concat(child.postorder()); } return result.concat([this.value]); } }帮我修改这段代码,并且增加修改和查找
好的,根据你的要求,我给出修改后的代码,并增加了修改和查找方法:
```javascript
class Tree {
constructor(value) {
this.value = value;
this.children = [];
}
addChild(value) {
this.children.push(new Tree(value));
}
inorder() {
if (!this.children.length) return [this.value];
let result = [];
for (let child of this.children) {
result = result.concat(child.inorder());
}
return [this.value].concat(result);
}
preorder() {
let result = [this.value];
for (let child of this.children) {
result = result.concat(child.preorder());
}
return result;
}
postorder() {
if (!this.children.length) return [this.value];
let result = [];
for (let child of this.children) {
result = result.concat(child.postorder());
}
return result.concat([this.value]);
}
modifyValue(newValue) {
this.value = newValue;
}
findNode(value) {
if (this.value === value) return this;
for (let child of this.children) {
const result = child.findNode(value);
if (result) return result;
}
return null;
}
}
```
在这个修改后的代码中,我添加了 `modifyValue(newValue)` 和 `findNode(value)` 方法。
`modifyValue(newValue)` 方法可以用于修改树节点的值,你只需要调用该方法并传入新的值即可。
`findNode(value)` 方法可以用于查找树中某个特定的节点,你只需要传入要查找的值,该方法会返回该节点的引用。如果没有找到该节点,则返回 `null`。
js 遍历 tree
### 回答1:
在 JavaScript 中遍历树的常见方法有两种:深度优先搜索和广度优先搜索。
对于深度优先搜索,通常使用递归的方式遍历每个子树。例如:
```
function traverse(node) {
console.log(node.value);
for (let child of node.children) {
traverse(child);
}
}
```
对于广度优先搜索,通常使用队列的数据结构。例如:
```
function traverse(node) {
let queue = [node];
while (queue.length > 0) {
let current = queue.shift();
console.log(current.value);
for (let child of current.children) {
queue.push(child);
}
}
}
```
希望这些内容能帮到你!
### 回答2:
在Javascript中,遍历树(Tree)是一种常见的操作,以下我将介绍三种常见的树遍历方法。
1. 深度优先遍历(DFS):
深度优先遍历是一种先遍历子节点,再遍历兄弟节点的方法。对于一个节点,先输出当前节点的值,然后依次遍历其子节点,直到所有子节点都被遍历完毕,再遍历该节点的兄弟节点。这种方法递归地从根节点开始遍历整个树。
2. 广度优先遍历(BFS):
广度优先遍历是一种先遍历同一层级的节点,再遍历下一层级节点的方法。对于一个节点,先输出当前节点的值,然后遍历该节点的所有子节点,再按顺序遍历子节点的子节点,以此类推,直到遍历完整棵树。这种方法通常使用队列来实现。
3. 前序遍历、中序遍历和后序遍历:
前序遍历(Pre-order)、中序遍历(In-order)和后序遍历(Post-order)是针对二叉树的遍历方法。前序遍历先输出当前节点的值,再依次遍历左子树和右子树;中序遍历先遍历左子树,然后输出当前节点的值,最后遍历右子树;后序遍历先遍历左子树和右子树,最后输出当前节点的值。这三种遍历方法也可以用来遍历一般的树结构,只需要根据需要自行定义遍历顺序。
以上是常用的树遍历方法,通过这些方法可以遍历树的所有节点,并根据需要进行相应的操作。在实际应用中,根据不同的场景和需求选择合适的遍历方式能够更高效地处理树结构的数据。
### 回答3:
在JavaScript中遍历树状结构(tree)通常使用递归函数来完成。下面是一个示例代码:
```javascript
// 定义树节点类
class Node {
constructor(value) {
this.value = value;
this.children = [];
}
addChild(node) {
this.children.push(node);
}
}
// 遍历树的递归函数
function traverseTree(node) {
console.log(node.value); // 输出当前节点的值
for (let i = 0; i < node.children.length; i++) {
traverseTree(node.children[i]); // 递归遍历子节点
}
}
// 创建一个树状结构
const root = new Node(1);
const child1 = new Node(2);
const child2 = new Node(3);
const grandchild1 = new Node(4);
const grandchild2 = new Node(5);
root.addChild(child1);
root.addChild(child2);
child1.addChild(grandchild1);
child1.addChild(grandchild2);
// 遍历树
traverseTree(root);
```
上述代码中,我们首先定义了一个树节点类 `Node`,每个节点有一个值以及一个子节点数组。然后定义了 `addChild` 方法用于向节点添加子节点。
接下来,我们定义了 `traverseTree` 递归函数,它通过打印当前节点的值来实现遍历树的功能。在函数中,我们首先打印当前节点的值,然后循环遍历当前节点的子节点,并对每个子节点递归调用 `traverseTree` 函数。
最后,我们创建一个树状结构,并调用 `traverseTree` 函数来遍历这个树。
希望以上示例能够帮助你理解如何使用JavaScript来遍历树状结构。
阅读全文
相关推荐
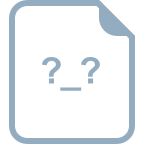
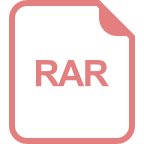
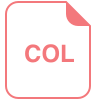
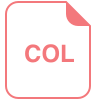
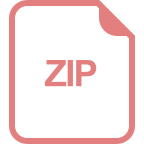
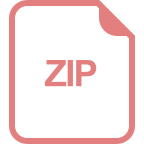
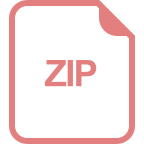
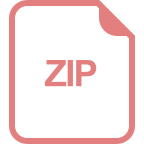
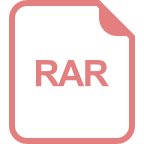
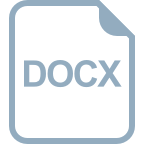
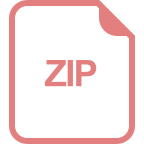
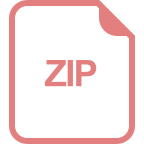