编写一个Java程序,包含类Acount、CheckingAccount、Main,其中Main已经实现,请你编写Acount和CheckingAccount类。 (1)编写一个类Account表示普通账户对象,包含以下成员
时间: 2024-03-16 07:42:18 浏览: 110
Account类的代码如下:
```java
public class Account {
private String owner; // 账户拥有者
private double balance; // 账户余额
// 构造方法
public Account(String owner, double balance) {
this.owner = owner;
this.balance = balance;
}
// 存款
public void deposit(double amount) {
balance += amount;
}
// 取款
public void withdraw(double amount) throws InsufficientFundsException {
if (balance < amount) {
throw new InsufficientFundsException();
}
balance -= amount;
}
// 获取账户余额
public double getBalance() {
return balance;
}
// 获取账户拥有者
public String getOwner() {
return owner;
}
// 设置账户拥有者
public void setOwner(String owner) {
this.owner = owner;
}
}
```
CheckingAccount类继承自Account类,代表一个支票账户对象,包含以下成员:
- overdraftProtection:透支保护金额
CheckingAccount类的代码如下:
```java
public class CheckingAccount extends Account {
private double overdraftProtection; // 透支保护金额
// 构造方法
public CheckingAccount(String owner, double balance, double overdraftProtection) {
super(owner, balance);
this.overdraftProtection = overdraftProtection;
}
// 取款
@Override
public void withdraw(double amount) throws InsufficientFundsException {
if (getBalance() + overdraftProtection < amount) {
throw new InsufficientFundsException();
}
if (getBalance() >= amount) {
super.withdraw(amount);
} else {
double overdraft = amount - getBalance();
super.withdraw(getBalance());
overdraftProtection -= overdraft;
}
}
// 获取透支保护金额
public double getOverdraftProtection() {
return overdraftProtection;
}
// 设置透支保护金额
public void setOverdraftProtection(double overdraftProtection) {
this.overdraftProtection = overdraftProtection;
}
}
```
InsufficientFundsException是一个自定义异常类,表示余额不足的错误,代码如下:
```java
public class InsufficientFundsException extends Exception {
public InsufficientFundsException() {
super("Insufficient funds in your account!");
}
}
```
阅读全文
相关推荐
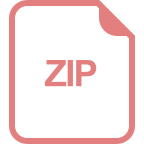
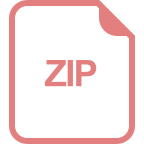
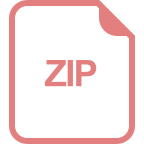















