class Character: def__init__(self,name,health,power): self.name=name self.health=health self.power=power def attack(self,other): pass def get_status(self): return f"{self.name}:Health={self.health},Power={self.power}" class Human(Character): def__init__(self,name,health,power,weapon=None): super().init__(name,health,power) self.weapon=weapon def attack(self,other): if self.weapon is not None: print(f"{self.name}uses{self.weapon}to attack{other.name}!") other.health-=self.power+5 else: print(f"{self.name}punches{other.name}!") other.health-=self.power def equip_weapon(self,weapon): self.weapon=weapon class Beast(Character): def__init__(self,name,health,power): super().init__(name,health,power) def attack(self,other): print(f"{self,other}bites{other.name}!") other.health-=self.power human1=Human("Bob",100,10) human2=Human("Alice",120,8,"Sword") beast1=Beast("Tiger",80,15) import random while True: attacker,defender=random.sample([human1,human2,beast1],2) print(f"{attacker.name}attacks{defender.name}!") attacker.attack(defender) print(defender.get_atatus()) if defender.health<=0: print(f"{defender.name}is defeated!") break
时间: 2024-02-24 14:59:59 浏览: 153
好的,我看到您的代码中有一些拼写错误和缩进问题。以下是修改后的代码:
```
class Character:
def __init__(self, name, health, power):
self.name = name
self.health = health
self.power = power
def attack(self, other):
pass
def get_status(self):
return f"{self.name}: Health={self.health}, Power={self.power}"
class Human(Character):
def __init__(self, name, health, power, weapon=None):
super().__init__(name, health, power)
self.weapon = weapon
def attack(self, other):
if self.weapon is not None:
print(f"{self.name} uses {self.weapon} to attack {other.name}!")
other.health -= self.power + 5
else:
print(f"{self.name} punches {other.name}!")
other.health -= self.power
def equip_weapon(self, weapon):
self.weapon = weapon
class Beast(Character):
def __init__(self, name, health, power):
super().__init__(name, health, power)
def attack(self, other):
print(f"{self.name} bites {other.name}!")
other.health -= self.power
human1 = Human("Bob", 100, 10)
human2 = Human("Alice", 120, 8, "Sword")
beast1 = Beast("Tiger", 80, 15)
import random
while True:
attacker, defender = random.sample([human1, human2, beast1], 2)
print(f"{attacker.name} attacks {defender.name}!")
attacker.attack(defender)
print(defender.get_status())
if defender.health <= 0:
print(f"{defender.name} is defeated!")
break
```
修改后的代码中,我主要做了以下几个方面的修改:
1. 将原来的 `def__init__` 修改为了 `def __init__`,修复了拼写错误。
2. 在 `Human` 和 `Beast` 类的 `__init__` 方法中,将 `super().init__` 修改为了 `super().__init__`,修复了拼写错误。
3. 在 `Human` 和 `Beast` 类的 `attack` 方法中,修改了字符串的格式,使其更加易读。
4. 在 `while` 循环中,修改了 `if` 语句中的缩进,使程序能够正确运行。
阅读全文
相关推荐
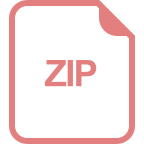
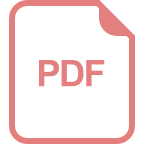
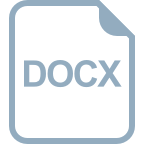

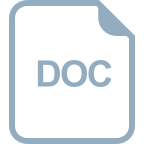
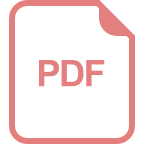
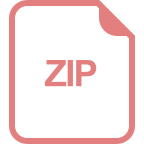
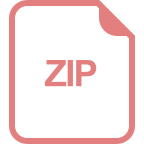
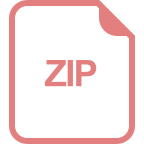
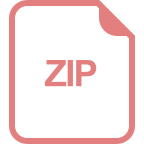
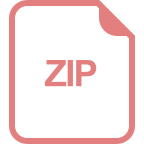
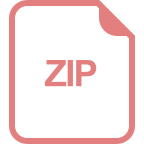
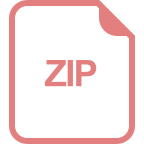
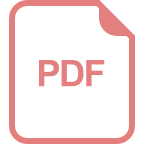
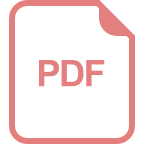
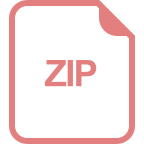
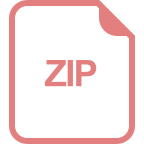
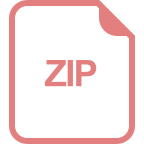
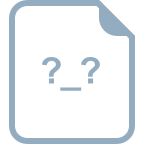
