C语言中使用链表代替std::map
时间: 2023-07-20 15:43:49 浏览: 151
在C语言中,可以使用链表来代替std::map来进行键-值对的存储和查找。
具体实现可以使用一个结构体来表示每个键值对,包括一个指向下一个结构体的指针和键值对的键和值。
例如:
```
typedef struct node {
char *key;
int value;
struct node *next;
} Node;
Node *head = NULL;
// 插入键值对
void insert(char *key, int value) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->key = key;
newNode->value = value;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
} else {
Node *current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 查找键值对
int find(char *key) {
Node *current = head;
while (current != NULL) {
if (strcmp(current->key, key) == 0) {
return current->value;
}
current = current->next;
}
return -1;
}
```
这样就可以使用链表来存储和查找键值对了。需要注意的是,在插入时应该检查是否已经存在相同的键,如果存在则更新对应的值。
阅读全文
相关推荐
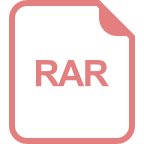
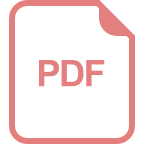
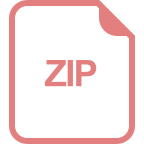
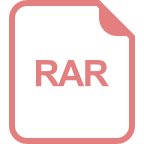
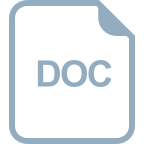
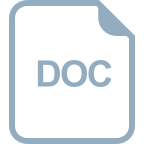
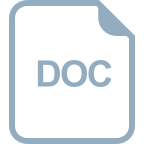
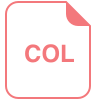
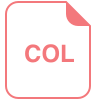
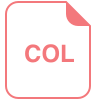
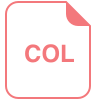
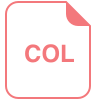
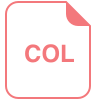
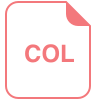
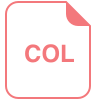