请帮我把线程池或异步编程框架加进去
时间: 2024-04-22 07:27:53 浏览: 13
下是一个示例代码,将线程池(使用`pool`库)和异步编程框架(使用`libev`库)结合起来,实现同时处理多个客户端连接的功能。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <pthreadpool.h>
#include <ev.h>
#define MAX_CLIENTS 10
#define SERVER_PORT 8080
typedef struct {
int client_fd;
struct sockaddr_in client_addr;
} ClientInfo;
void PerformServerTransfer(int client_fd) {
char buffer[1024];
memset(buffer, 0, sizeof(buffer));
// 接收客户端消息
ssize_t bytes = recv(client_fd, buffer, sizeof(buffer), 0);
if (bytes <= 0) {
printf("Error receiving message from client %d\n", client_fd);
return;
}
// 处理客户端的消息
printf("Received message from client %d: %s\n", client_fd, buffer);
// 在这里根据消息包进行相应的处理
// ...
// 发送响应给客户端
send(client_fd, "Message received", strlen("Message received"), 0);
}
void handleClient(ev_io *watcher, int revents) {
if (EV_ERROR & revents) {
printf("Error event\n");
return;
}
int client_fd = watcher->fd;
PerformServerTransfer(client_fd);
// 关闭客户端套接字
close(client_fd);
free(watcher);
}
void acceptClient(ev_io *watcher, int revents) {
if (EV_ERROR & revents) {
printf("Error event\n");
return;
}
int server_fd = watcher->fd;
struct sockaddr_in client_addr;
socklen_t addrlen = sizeof(client_addr);
int client_fd = accept(server_fd, (struct sockaddr *)&client_addr, &addrlen);
if (client_fd < 0) {
printf("Accept error\n");
return;
}
printf("New connection. Socket fd: %d, IP: %s, Port: %d\n",
client_fd, inet_ntoa(client_addr.sin_addr), ntohs(client_addr.sin_port));
// 创建并初始化一个ev_io,用于监听客户端套接字上的读事件
ev_io *client_watcher = (ev_io*)malloc(sizeof(ev_io));
ev_io_init(client_watcher, handleClient, client_fd, EV_READ);
ev_io_start(EV_DEFAULT_ client_watcher);
}
void pthreadServerListening() {
int server_fd;
struct sockaddr_in server_addr;
// 创建服务器套接字
if ((server_fd = socket(AF_INET, SOCK_STREAM, 0)) == -1) {
perror("Socket creation failed");
exit(EXIT_FAILURE);
}
// 设置服务器地址和端口
memset(&server_addr, 0, sizeof(server_addr));
server_addr.sin_family = AF_INET;
server_addr.sin_addr.s_addr = INADDR_ANY;
server_addr.sin_port = htons(SERVER_PORT);
// 绑定服务器套接字到指定地址和端口
if (bind(server_fd, (struct sockaddr *)&server_addr, sizeof(server_addr)) == -1) {
perror("Binding failed");
exit(EXIT_FAILURE);
}
// 监听连接
if (listen(server_fd, 5) == -1) {
perror("Listening failed");
exit(EXIT_FAILURE);
}
printf("Server started. Waiting for incoming connections...\n");
// 创建线程池
pthreadpool_t pool = pthreadpool_create(0);
// 创建一个ev_io,用于监听服务器套接字上的读事件
struct ev_loop *loop = EV_DEFAULT;
ev_io server_watcher;
ev_io_init(&server_watcher, acceptClient, server_fd, EV_READ);
ev_io_start(loop, &server_watcher);
// 进入事件循环
ev_run(loop, 0);
// 销毁线程池
pthreadpool_destroy(pool);
// 关闭服务器套接字
close(server_fd);
}
int main() {
pthreadServerListening();
return 0;
}
```
在上述代码中,我们使用了`libev`库来实现异步事件循环。当有新的客户端连接时,会创建一个新的`ev_io`对象来监听客户端套接字上的读事件,并将其加入事件循环。每当有读事件发生时,会调用`handleClient`函数来处理客户端的消息。
同时,我们使用了`pthreadpool`库来创建线程池。在`pthreadServerListening`函数中,我们首先创建了线程池,然后在事件循环中,每当有新的客户端连接时,将处理该连接的任务提交给线程池中的一个线程进行处理。
请注意,以上代码只是一个示例,使用线程池和异步编程框架需要根据实际情况进行配置和优化。在实际使用中,还需注意线程安全和资源管理等问题。
相关推荐
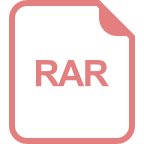
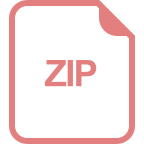














